模拟退火算法java
时间: 2023-10-23 19:13:45 浏览: 45
模拟退火算法是一种用于解决优化问题的启发式算法。它通过模拟金属退火过程中的原子的行为,以寻找目标函数的全局最优解。算法从一个较高的温度出发,并逐渐降低温度参数,期间以一定概率跳出局部最优解,以增加找到全局最优解的可能性。
在Java中,可以使用以下步骤来实现模拟退火算法:
1. 定义目标函数:首先需要定义一个目标函数,该函数的最大值或最小值是我们希望找到的全局最优解。
2. 初始化温度和初始解:选择一个适当的初始解,并确定初始温度。
3. 迭代过程:在每次迭代中,通过随机生成新解或对当前解进行微小的改变来生成下一个解。根据目标函数的变化和当前温度,决定是否接受新解。如果新解更好或接近全局最优解,那么它将被接受。如果新解不如当前解好,以一定概率仍然可能接受它,这是为了避免陷入局部最优解。
4. 降温过程:随着迭代的进行,温度会逐渐下降,这有助于控制接受新解的概率。可以使用不同的降温策略,例如线性降温或指数降温,根据问题的特性选择合适的降温方式。
5. 终止条件:算法的终止条件可以是达到一定的迭代次数或满足一定的停止准则,例如目标函数的变化小于某个阈值。
以下是一个简单的示例代码,演示了如何在Java中实现模拟退火算法:
```
// 定义目标函数
public double calculateObjectiveFunction(double x) {
// 根据问题定义目标函数
// 例如:return Math.sin(x);
}
// 模拟退火算法
public double simulatedAnnealing() {
double initialTemperature = 100.0; // 初始温度
double coolingRate = 0.01; // 降温率
double currentSolution = generateInitialSolution(); // 初始解
double bestSolution = currentSolution; // 当前最好的解
while (initialTemperature > 1.0) {
for (int i = 0; i < numIterations; i++) {
double newSolution = generateNewSolution(currentSolution); // 生成新解
double currentObjective = calculateObjectiveFunction(currentSolution);
double newObjective = calculateObjectiveFunction(newSolution);
double acceptanceProbability = calculateAcceptanceProbability(currentObjective, newObjective, initialTemperature);
if (acceptanceProbability > Math.random()) {
currentSolution = newSolution; // 接受新解
}
if (currentObjective > calculateObjectiveFunction(bestSolution)) {
bestSolution = currentSolution; // 更新最好的解
}
}
initialTemperature *= 1 - coolingRate; // 降温
}
return calculateObjectiveFunction(bestSolution); // 返回最优解对应的目标函数值
}
// 计算接受新解的概率
public double calculateAcceptanceProbability(double currentObjective, double newObjective, double temperature) {
if (newObjective > currentObjective) {
return 1.0; // 新解更好,概率为1,直接接受
} else {
return Math.exp((newObjective - currentObjective) / temperature);
}
}
// 生成初始解
public double generateInitialSolution() {
// 根据问题的要求生成一个初始解
}
// 生成新解
public double generateNewSolution(double currentSolution) {
// 根据问题的要求生成一个新解
}
// 示例相关问题:
相关推荐
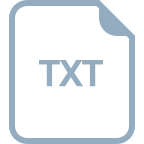














