模拟退火算法代码java
时间: 2024-09-21 08:00:39 浏览: 34
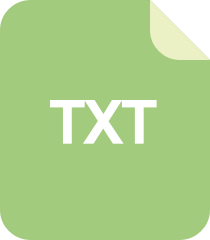
模拟退火算法+java实现+优化问题
模拟退火算法是一种全局优化搜索技术,常用于解决复杂的问题,如组合优化、机器学习等。在Java中实现模拟退火的过程可以分为以下几个步骤:
```java
import java.util.Random;
public class SimulatedAnnealing {
private double initialTemperature; // 初始温度
private double coolingRate; // 冷却速率
private int maxIterations; // 最大迭代次数
private double currentSolution; // 当前解决方案
private double bestSolution; // 最佳解决方案
// 初始化算法参数
public SimulatedAnnealing(double initialTemp, double coolingRate, int iterations) {
this.initialTemperature = initialTemp;
this.coolingRate = coolingRate;
this.maxIterations = iterations;
}
// 定义目标函数(这里假设是一个简单的平方误差)
private double objectiveFunction(double solution) {
return Math.pow(solution - targetValue, 2);
}
// 主循环
public void run() {
Random rand = new Random();
for (int i = 0; i < maxIterations; i++) {
double newSolution = proposeNewSolution(rand); // 提出新解
double energyGain = objectiveFunction(newSolution) - objectiveFunction(currentSolution);
if (energyGain <= 0 || acceptWithProbability(energyGain)) { // 如果接受新解或满足随机概率,则更新当前最佳和当前状态
currentSolution = newSolution;
if (newSolution < bestSolution || (newSolution == bestSolution && rand.nextDouble() < 0.5)) { // 随机接受平局
bestSolution = newSolution;
}
}
currentTemperature *= coolingRate; // 降低温度
}
}
// 新解提案策略
private double proposeNewSolution(Random rand) {
// 实现具体的新解提案策略,比如随机扰动当前解
return currentSolution + rand.nextDouble() * range;
}
// 接受新解的概率计算
private boolean acceptWithProbability(double energyGain) {
return Math.exp(-energyGain / currentTemperature) > rand.nextDouble(); // Metropolis-Hastings准则
}
// ...其他辅助方法和变量定义...
// 示例运行
public static void main(String[] args) {
SimulatedAnnealing algo = new SimulatedAnnealing(100.0, 0.95, 1000);
algo.run();
System.out.println("Best Solution found: " + algo.bestSolution);
}
}
```
这是模拟退火的一个基础版示例,实际应用中你需要根据具体问题调整`objectiveFunction`和`proposeNewSolution`的具体实现。
阅读全文
相关推荐
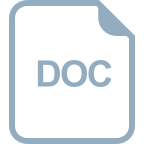
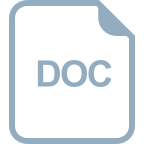
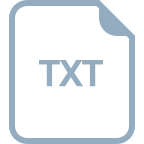
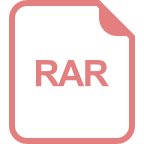




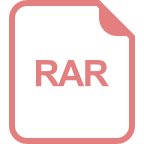
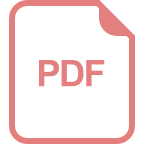
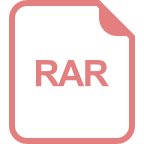
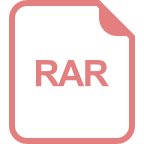
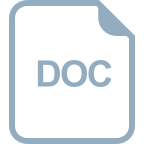



