python数据框中如果最大值索引不止一个,怎么取最后一个的索引
时间: 2024-04-13 16:28:49 浏览: 40
你可以使用 `numpy` 库中的 `argmax` 函数来获取最大值的索引。然后,你可以使用 `[::-1]` 的切片操作将索引列表反转,然后取第一个索引值即可。以下是一个示例代码:
```python
import numpy as np
# 假设你的数据框名为 df,列名为 column_name
column_name = 'column_name'
max_index = np.argmax(df[column_name].values)
# 取最后一个最大值索引
last_max_index = max_index[::-1][0]
```
通过这种方式,你可以获取到最大值的最后一个索引。请确保你已经导入了 `numpy` 库。
相关问题
python获取向量中最大值及其索引,最大值不只一个
要获取向量中最大值及其索引,可以使用numpy库中的argmax函数。如果最大值不只一个,可以使用numpy库中的where函数来获取所有最大值的索引。以下是示例代码:
```python
import numpy as np
# 定义一个向量
vec = np.array([0, 3, 1, 5, 7, 5])
# 获取最大值及其索引
max_val = np.max(vec)
max_idx = np.argmax(vec)
print("最大值:", max_val)
print("最大值索引:", max_idx)
# 获取所有最大值的索引
max_idxs = np.where(vec == np.max(vec))[0]
print("所有最大值索引:", max_idxs)
```
输出结果为:
```
最大值: 7
最大值索引: 4
所有最大值索引: [4 5]
```
其中,`np.max()`函数用于获取向量中的最大值,`np.argmax()`函数用于获取最大值的索引,`np.where()`函数用于获取满足条件的索引。注意,`np.where()`函数返回的是一个元组,因此需要使用索引 `[0]` 来获取索引数组。
用Python编写一个程序,找到列表中的最大值,并打印出最大值及其索引
好的,下面是一个示例程序:
```python
import random
# 生成一个包含10个随机整数的列表
my_list = [random.randint(1, 100) for _ in range(10)]
print("原始列表:", my_list)
# 初始化最大值和最大值索引为列表第一个元素
max_value = my_list[0]
max_index = 0
# 遍历列表中的所有元素
for i in range(1, len(my_list)):
if my_list[i] > max_value:
# 如果当前元素比最大值大,则更新最大值和最大值索引
max_value = my_list[i]
max_index = i
# 打印最大值及其索引
print("最大值为", max_value, ",索引为", max_index)
```
这个程序首先生成一个包含10个随机整数的列表,然后遍历整个列表,找到最大值及其索引。最后打印出最大值及其索引。您可以将`my_list`变量替换为您自己的列表来使用这个程序。
相关推荐
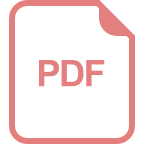
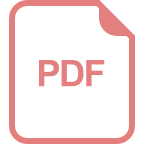
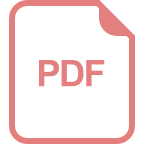












