实现这九个功能<1>创建(带头尾插) <2>指定学号前插入 <3>按学号删除 <4>计算学生总数 <5>按学号查找 <6>按姓名查找 <7>显示所有学生 <8>删除指定成绩的学生 <9>退出程序
时间: 2024-10-27 19:11:38 浏览: 15
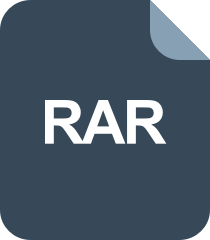
test3-插入.rar_尾插法_有头插法_链表 插入创建
在C语言中,我们可以使用链表数据结构来实现上述功能。以下是相应的代码片段和描述:
1. **创建(带头尾插)**:
```c
struct Node {
int id;
char name[50];
float score;
struct Node* next;
};
void createList(struct Node** head) {
*head = malloc(sizeof(struct Node));
(*head)->id = 0;
(*head)->name[0] = '\0';
(*head)->score = 0.0;
(*head)->next = NULL;
}
```
2. **指定学号前插入**:
```c
void insertBeforeId(struct Node** head, int targetId, struct Node* newNode) {
struct Node* current = *head;
while (current->id != targetId && current->next != NULL) {
current = current->next;
}
if (current->id == targetId) {
newNode->next = current->next;
current->next = newNode;
} else {
printf("Target id not found.\n");
}
}
```
3. **按学号删除**:
```c
void deleteById(struct Node** head, int targetId) {
struct Node* current = *head;
struct Node* previous = NULL;
while (current != NULL) {
if (current->id == targetId) {
if (previous == NULL) {
*head = current->next;
} else {
previous->next = current->next;
}
free(current);
break;
}
previous = current;
current = current->next;
}
}
```
4. **计算学生总数**:
```c
int countStudents(struct Node* head) {
int count = 0;
struct Node* current = head;
while (current != NULL) {
count++;
current = current->next;
}
return count;
}
```
5. **按学号查找**:
```c
struct Node* findById(struct Node* head, int targetId) {
struct Node* current = head;
while (current != NULL) {
if (current->id == targetId) {
return current;
}
current = current->next;
}
return NULL;
}
```
6. **按姓名查找**:
```c
struct Node* findByName(struct Node* head, char* targetName) {
struct Node* current = head;
while (current != NULL) {
if (strcmp(current->name, targetName) == 0) {
return current;
}
current = current->next;
}
return NULL;
}
```
7. **显示所有学生**:
```c
void displayStudents(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("学号:%d 姓名:%s 成绩:%f\n", current->id, current->name, current->score);
current = current->next;
}
}
```
8. **删除指定成绩的学生**:
这个需求可能需要额外的数据结构如哈希表或排序数组,因为直接在链表上查找特定成绩的时间复杂度较高。可以先按成绩排序,然后遍历删除匹配的成绩。
9. **退出程序**:
```c
void exitProgram() {
printf("Exiting the program...\n");
exit(0);
}
```
以上是一些核心函数的简要实现,实际使用时还需要考虑错误处理和用户交互界面。你可以根据这些函数组合出完整程序,让使用者选择相应功能来操作。
阅读全文
相关推荐
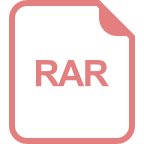
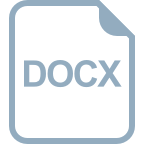
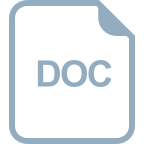
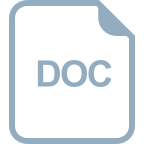
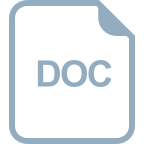
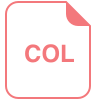


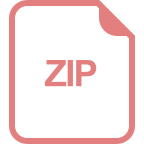
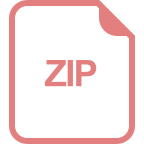
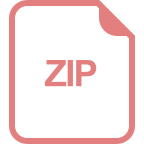
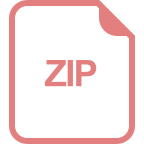
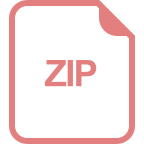