用C语言编写一个算法,删除一个队列中的一个指定元素e,写出完整代码
时间: 2023-06-08 17:04:51 浏览: 198
以下是用 C 语言编写删除队列中指定元素 e 的算法代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct queue
{
int front, rear;
int capacity;
int *arr;
} queue;
queue *createQueue(int capacity)
{
queue *q = (queue *)malloc(sizeof(queue));
q->front = q->rear = -1;
q->capacity = capacity;
q->arr = (int *)malloc(q->capacity * sizeof(int));
return q;
}
int isFull(queue *q)
{
return (q->rear == q->capacity - 1);
}
int isEmpty(queue *q)
{
return (q->front == -1 || q->front > q->rear);
}
void enqueue(queue *q, int data)
{
if (isFull(q))
{
printf("Queue is full.\n");
return;
}
else
{
q->arr[++q->rear] = data;
if (q->front == -1)
{
q->front = 0;
}
}
}
void dequeue(queue *q, int data)
{
if (isEmpty(q))
{
printf("Queue is empty.\n");
return;
}
else
{
int i;
for (i = q->front; i <= q->rear; i++)
{
if (q->arr[i] == data)
{
break;
}
}
if (i > q->rear)
{
printf("Element not found in queue.\n");
return;
}
for (; i < q->rear; i++)
{
q->arr[i] = q->arr[i + 1];
}
q->rear--;
if (q->front > q->rear)
{
q->front = q->rear = -1;
}
}
}
void display(queue *q)
{
if (isEmpty(q))
{
printf("Queue is empty.\n");
}
else
{
int i;
printf("Queue: ");
for (i = q->front; i <= q->rear; i++)
{
printf("%d ", q->arr[i]);
}
printf("\n");
}
}
int main()
{
int capacity, opt, data;
printf("Enter the capacity of the queue: ");
scanf("%d", &capacity);
queue *q = createQueue(capacity);
while (1)
{
printf("\nOptions:\n");
printf("1. Enqueue\n");
printf("2. Dequeue\n");
printf("3. Display\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &opt);
switch (opt)
{
case 1:
printf("Enter the data to enqueue: ");
scanf("%d", &data);
enqueue(q, data);
break;
case 2:
printf("Enter the data to dequeue: ");
scanf("%d", &data);
dequeue(q, data);
break;
case 3:
display(q);
break;
case 4:
exit(0);
default:
printf("Invalid option.\n");
}
}
return 0;
}
```
在上述代码中,我们先定义了一个结构体 `queue`,它包含了队列的属性和方法:`front` 表示队首下标,`rear` 表示队尾下标,`capacity` 表示队列容量,`arr` 表示数组指针,用于存储队列元素;`createQueue()` 用于创建队列实例,初始化队列属性;`isFull()` 和 `isEmpty()` 用于判断队列是否已满或为空;`enqueue()` 和 `dequeue()` 用于在队列中分别插入和删除元素;`display()` 用于打印队列元素。再在 `main()` 函数中,我们先从用户输入读取队列容量,然后根据用户选择调用不同的队列方法。在 `dequeue()` 方法中,我们通过遍历队列元素,找到指定元素在队列中的位置并删除。如果指定元素不存在于队列中,则提示“Element not found in queue.”。
阅读全文
相关推荐
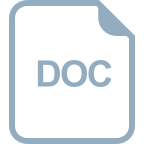
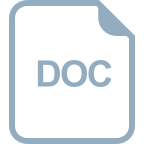
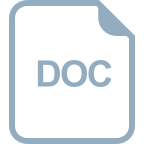













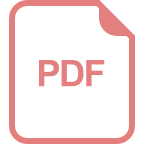

