用c语言编写程序,模拟实现扫描算法,并计算寻道距离。
时间: 2024-02-25 17:54:12 浏览: 57
好的,下面是用 C 语言实现扫描算法的代码,同时也计算了寻道距离:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_REQUESTS 100
void swap(int *a, int *b) {
int tmp = *a;
*a = *b;
*b = tmp;
}
void sort(int arr[], int n) {
for (int i = 0; i < n-1; i++) {
for (int j = i+1; j < n; j++) {
if (arr[i] > arr[j]) {
swap(&arr[i], &arr[j]);
}
}
}
}
int find_index(int arr[], int n, int value) {
for (int i = 0; i < n; i++) {
if (arr[i] == value) {
return i;
}
}
return -1;
}
void scan(int requests[], int n, int start, int end) {
int current = start;
int direction = 1;
int total_distance = 0;
int i = find_index(requests, n, current);
if (i < 0) {
printf("Invalid start position\n");
return;
}
sort(requests, n);
while (i >= 0 && i < n) {
printf("Moving to track %d\n", requests[i]);
total_distance += abs(current - requests[i]);
current = requests[i];
if (i == n-1) {
direction = -1;
}
i += direction;
}
total_distance += abs(current - end);
printf("Total distance traveled: %d\n", total_distance);
}
int main() {
int requests[MAX_REQUESTS];
int n, start, end;
printf("Enter number of requests: ");
scanf("%d", &n);
if (n > MAX_REQUESTS) {
printf("Maximum number of requests is %d\n", MAX_REQUESTS);
return 1;
}
printf("Enter requests: ");
for (int i = 0; i < n; i++) {
scanf("%d", &requests[i]);
}
printf("Enter start position: ");
scanf("%d", &start);
printf("Enter end position: ");
scanf("%d", &end);
scan(requests, n, start, end);
return 0;
}
```
在上面的代码中,使用了一个 scan() 函数来实现扫描算法。在函数中,首先找到当前位置在请求中的位置,然后对请求进行排序。接着,根据当前位置和请求位置的大小关系,确定电梯的运行方向,并计算出电梯需要移动的距离。最后,计算出电梯总共运行的距离。
主函数中,首先从用户输入中读取请求,起始位置和结束位置。然后,调用 scan() 函数来模拟电梯的运行过程,并输出结果。
注意:上述代码中未考虑输入数据的合法性和边界情况,你可以根据需要进行修改。
阅读全文
相关推荐
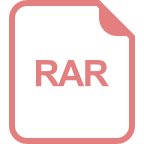


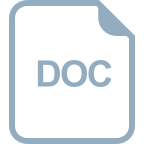
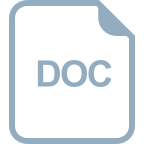
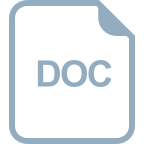





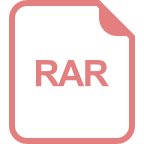
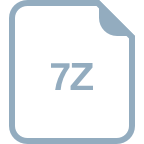
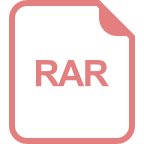
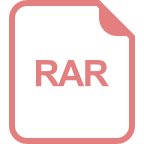
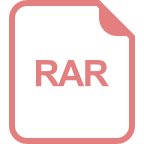