用c语言实现无损分解算法
时间: 2023-09-23 12:09:59 浏览: 45
无损分解算法也称为哈夫曼编码算法,是一种将数字数据压缩为更小的数据的技术。以下是用C语言实现无损分解算法的基本步骤:
1.定义结构体,用来存储字符及其频率信息。
```c
struct node
{
char ch;
int freq;
struct node *left, *right;
};
```
2.定义比较函数,用来将结构体按频率从小到大排序。
```c
int compare(const void* a, const void* b)
{
struct node *x = (struct node*)a;
struct node *y = (struct node*)b;
return (x->freq - y->freq);
}
```
3.定义函数,用来创建哈夫曼树。
```c
struct node* create_huffman_tree(char data[], int freq[], int len)
{
struct node *left, *right, *top;
struct node* nodes = (struct node*)malloc(len * sizeof(struct node));
for (int i = 0; i < len; i++)
{
nodes[i].ch = data[i];
nodes[i].freq = freq[i];
nodes[i].left = nodes[i].right = NULL;
}
qsort(nodes, len, sizeof(struct node), compare);
while (len > 1)
{
left = &nodes[0];
right = &nodes[1];
top = (struct node*)malloc(sizeof(struct node));
top->ch = '$';
top->freq = left->freq + right->freq;
top->left = left;
top->right = right;
nodes[0] = *top;
for (int i = 1; i < len - 1; i++)
nodes[i] = nodes[i + 1];
len--;
qsort(nodes, len, sizeof(struct node), compare);
}
return &nodes[0];
}
```
4.定义函数,用来打印哈夫曼编码。
```c
void print_huffman_codes(struct node* root, int arr[], int top)
{
if (root->left)
{
arr[top] = 0;
print_huffman_codes(root->left, arr, top + 1);
}
if (root->right)
{
arr[top] = 1;
print_huffman_codes(root->right, arr, top + 1);
}
if (!root->left && !root->right)
{
printf("%c: ", root->ch);
for (int i = 0; i < top; i++)
printf("%d", arr[i]);
printf("\n");
}
}
```
5.定义主函数,调用以上函数实现无损分解算法。
```c
int main()
{
char data[] = { 'a', 'b', 'c', 'd', 'e', 'f' };
int freq[] = { 5, 9, 12, 13, 16, 45 };
int len = sizeof(data) / sizeof(data[0]);
struct node* root = create_huffman_tree(data, freq, len);
int arr[100], top = 0;
print_huffman_codes(root, arr, top);
return 0;
}
```
以上就是用C语言实现无损分解算法的基本步骤。
相关推荐
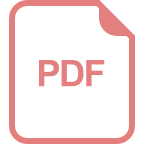
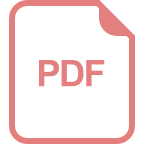














