qt怎么用opencv将图像显示为荧光效果
时间: 2023-05-21 18:07:19 浏览: 78
您可以使用 OpenCV 的颜色空间转换函数将图像转换为 HSV 颜色空间。然后可以调整图像的亮度和饱和度,以进一步增强荧光效果。最后,您可以将图像转换回 BGR 颜色空间,然后使用 Qt 的 QImage 和 QPainter 类来绘制图像并在应用程序中显示它们。以下是一些示例代码:
```
#include <opencv2/opencv.hpp>
#include <QImage>
#include <QPainter>
using namespace cv;
// Function to convert OpenCV Mat to QImage
QImage matToQImage(const Mat& mat)
{
// Convert the color format to RGB
Mat temp;
cvtColor(mat, temp, COLOR_BGR2RGB);
// Create a QImage from the OpenCV Mat data
QImage qImage(temp.data, temp.cols, temp.rows, temp.step, QImage::Format_RGB888);
qImage.bits(); // Force deep copy of data
return qImage;
}
// Function to convert QImage to OpenCV Mat
Mat qImageToMat(const QImage& qImage)
{
Mat mat(qImage.height(), qImage.width(), CV_8UC4, (uchar*)qImage.bits(), qImage.bytesPerLine());
cvtColor(mat, mat, COLOR_BGRA2BGR);
return mat;
}
// Function to apply fluorescent effect to an image
Mat applyFluorescentEffect(const Mat& src)
{
Mat hsv;
cvtColor(src, hsv, COLOR_BGR2HSV);
// Increase brightness and saturation to enhance the fluorescent effect
hsv.convertTo(hsv, -1, 1.5, 1.5);
Mat result;
cvtColor(hsv, result, COLOR_HSV2BGR);
return result;
}
int main()
{
// Load an image using OpenCV
Mat src = imread("path/to/image.jpg");
// Apply the fluorescent effect
Mat fluorescentImg = applyFluorescentEffect(src);
// Convert the OpenCV Mat to QImage
QImage qImage = matToQImage(fluorescentImg);
// Create a QPixmap from the QImage for display in a QLabel
QPixmap pixmap = QPixmap::fromImage(qImage);
// Display the QPixmap in a QLabel
QLabel label;
label.setPixmap(pixmap);
label.show();
return 0;
}
```
相关推荐
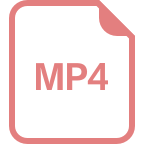
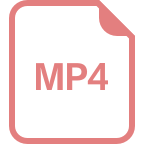
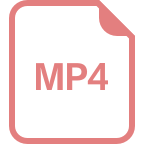














