python处理ansys有限元分析结果txt文件生成主应力轨迹线
时间: 2023-07-27 12:29:25 浏览: 73
要处理ANSYS有限元分析结果txt文件生成主应力轨迹线,可以使用Python中的numpy和matplotlib库。以下是一个简单的代码示例,可以帮助你开始:
```python
import numpy as np
import matplotlib.pyplot as plt
# 读取txt文件中的应力数据
with open('your_txt_file.txt', 'r') as f:
lines = f.readlines()
# 获取应力数据的行数和列数
num_rows = len(lines)
num_cols = len(lines[0].split())
# 将应力数据存储到numpy数组中
stress_data = np.zeros((num_rows, num_cols))
for i in range(num_rows):
stress_data[i] = np.array([float(x) for x in lines[i].split()])
# 循环计算每个节点的主应力轨迹线
for i in range(num_rows):
stress_tensor = np.array([[stress_data[i][0], stress_data[i][3], stress_data[i][4]],
[stress_data[i][3], stress_data[i][1], stress_data[i][5]],
[stress_data[i][4], stress_data[i][5], stress_data[i][2]]])
eig_vals, eig_vecs = np.linalg.eig(stress_tensor)
sorted_indices = np.argsort(eig_vals)[::-1]
eig_vals = eig_vals[sorted_indices]
eig_vecs = eig_vecs[:, sorted_indices]
r = np.sqrt((eig_vals[0] ** 2 + eig_vals[1] ** 2) / 2)
theta = np.linspace(0, 2 * np.pi, 100)
s1 = r + np.sqrt((r ** 2) * ((eig_vecs[0][0] * np.cos(theta) + eig_vecs[1][0] * np.sin(theta)) ** 2) + ((eig_vecs[0][1] * np.cos(theta) + eig_vecs[1][1] * np.sin(theta)) ** 2))
s2 = r - np.sqrt((r ** 2) * ((eig_vecs[0][0] * np.cos(theta) + eig_vecs[1][0] * np.sin(theta)) ** 2) + ((eig_vecs[0][1] * np.cos(theta) + eig_vecs[1][1] * np.sin(theta)) ** 2))
# 绘制主应力轨迹线
fig, ax = plt.subplots()
ax.plot(s1 * np.cos(theta), s1 * np.sin(theta), 'r-', label='s1')
ax.plot(s2 * np.cos(theta), s2 * np.sin(theta), 'b-', label='s2')
ax.legend()
ax.set_aspect('equal')
plt.show()
```
这段代码将读取指定的txt文件,获取应力数据的行数和列数,然后循环计算每个节点的主应力轨迹线,并将其绘制出来。你可以根据自己的需求修改这段代码,以便处理和绘制你所需的主应力轨迹线。
相关推荐
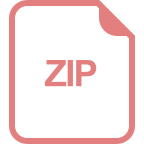
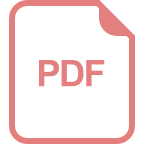














