14.职工信息查询程序 功能要求: 设计职工类,职工信息包括职工号、姓名、性别、年龄、学历、工资、住址、电话等 (自定义)(职工号不重复)。编写一个Java应用程序,实现职工信息从文件staff.txt读取并通过姓名、工号查找指定职工信息。
时间: 2023-12-06 16:04:30 浏览: 80
好的,根据你的要求,这是一个简单的职工信息查询程序实现:
首先,我们需要定义职工类,包含职工号、姓名、性别、年龄、学历、工资、住址、电话等属性。同时,需要覆盖equals和hashCode方法,确保职工号不重复。
```java
public class Staff {
private int id;
private String name;
private String gender;
private int age;
private String education;
private double salary;
private String address;
private String phone;
public Staff(int id, String name, String gender, int age, String education, double salary, String address, String phone) {
this.id = id;
this.name = name;
this.gender = gender;
this.age = age;
this.education = education;
this.salary = salary;
this.address = address;
this.phone = phone;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public int getAge() {
return age;
}
public String getEducation() {
return education;
}
public double getSalary() {
return salary;
}
public String getAddress() {
return address;
}
public String getPhone() {
return phone;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Staff staff = (Staff) o;
return id == staff.id;
}
@Override
public int hashCode() {
return Objects.hash(id);
}
}
```
接下来,我们需要读取staff.txt文件中的职工信息,并将其存储在一个列表中。
```java
public class StaffManager {
private List<Staff> staffList = new ArrayList<>();
public StaffManager() {
try {
BufferedReader reader = new BufferedReader(new FileReader("staff.txt"));
String line;
while ((line = reader.readLine()) != null) {
String[] fields = line.split(",");
int id = Integer.parseInt(fields[0]);
String name = fields[1];
String gender = fields[2];
int age = Integer.parseInt(fields[3]);
String education = fields[4];
double salary = Double.parseDouble(fields[5]);
String address = fields[6];
String phone = fields[7];
Staff staff = new Staff(id, name, gender, age, education, salary, address, phone);
staffList.add(staff);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public Staff findById(int id) {
for (Staff staff : staffList) {
if (staff.getId() == id) {
return staff;
}
}
return null;
}
public Staff findByName(String name) {
for (Staff staff : staffList) {
if (staff.getName().equals(name)) {
return staff;
}
}
return null;
}
}
```
最后,我们可以通过调用StaffManager类中的findById或findByName方法,来查找指定职工的信息。
```java
public static void main(String[] args) {
StaffManager manager = new StaffManager();
Scanner scanner = new Scanner(System.in);
System.out.println("请输入要查询的职工编号:");
int id = scanner.nextInt();
Staff staff = manager.findById(id);
if (staff != null) {
System.out.println(staff.getName() + "的信息如下:");
System.out.println("职工号:" + staff.getId());
System.out.println("姓名:" + staff.getName());
System.out.println("性别:" + staff.getGender());
System.out.println("年龄:" + staff.getAge());
System.out.println("学历:" + staff.getEducation());
System.out.println("工资:" + staff.getSalary());
System.out.println("住址:" + staff.getAddress());
System.out.println("电话:" + staff.getPhone());
} else {
System.out.println("没有找到职工编号为" + id + "的职工。");
}
}
```
当然,我们也可以通过调用findByName方法,来通过职工姓名查找职工信息。
阅读全文
相关推荐









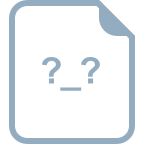








