定义一个结构体变量,其成员包括职工姓名,职工号,性别,工资,婚姻状况和家庭住址
时间: 2024-03-14 15:44:44 浏览: 119
好的,以下是一个定义职工信息的结构体变量的示例代码:
```c
struct Employee {
char name[50]; // 职工姓名
int id; // 职工号
char gender; // 性别
float salary; // 工资
char marital_status[10]; // 婚姻状况
char address[100]; // 家庭住址
};
```
这个结构体变量包含了职工的姓名、职工号、性别、工资、婚姻状况和家庭住址等信息。你可以根据需要修改成员的数据类型和名称。
相关问题
C语言程序定义一个结构体变量,其成员包括工姓名、职工号、性别、工资、婚姻状况和家庭地址"。
可以使用以下的C语言代码来定义一个包含上述成员的结构体变量:
```
struct Employee {
char name[50];
int id;
char gender;
float salary;
char marital_status[10];
char address[100];
};
```
其中,`struct Employee` 表示结构体的名称,可以根据需要自行修改。结构体中的每个成员都有自己的数据类型和名称,例如,`name` 是一个包含50个字符的字符串数组,`id` 是一个整型变量,`salary` 是一个浮点型变量,以此类推。在使用这个结构体时,可以通过以下方式来定义结构体变量:
```
struct Employee employee1;
employee1.id = 12345;
strcpy(employee1.name, "张三");
employee1.gender = 'M';
employee1.salary = 5000.0;
strcpy(employee1.marital_status, "已婚");
strcpy(employee1.address, "北京市朝阳区");
```
这里我们定义了一个名为 `employee1` 的结构体变量,并为其赋值。注意,在设置字符串类型的成员变量时,需要使用 `strcpy()` 函数来进行赋值操作。
c语言编写程序:定义一个职工结构体类型,定义职工结构体变量,从键盘输入一名职工 信息,然后输出。(假设职工信息包括:姓名、身份证号、工龄、工资)。
好的,以下是 C 语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
// 定义职工结构体类型
struct Employee {
char name[20];
char id[20];
int years;
float salary;
};
int main() {
struct Employee emp; // 定义职工结构体变量
// 从键盘输入职工信息
printf("请输入职工姓名:");
scanf("%s", emp.name);
printf("请输入职工身份证号:");
scanf("%s", emp.id);
printf("请输入职工工龄:");
scanf("%d", &emp.years);
printf("请输入职工工资:");
scanf("%f", &emp.salary);
// 输出职工信息
printf("职工姓名:%s\n", emp.name);
printf("职工身份证号:%s\n", emp.id);
printf("职工工龄:%d 年\n", emp.years);
printf("职工工资:%.2f 元\n", emp.salary);
return 0;
}
```
与 C++ 代码类似,运行程序后按照提示输入职工信息即可看到输出结果。需要注意的是,在 C 语言中,读取字符串需要使用 `%s` 格式控制符,而读取整数和浮点数需要加上 `&` 取地址符。
阅读全文
相关推荐
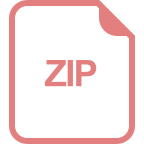
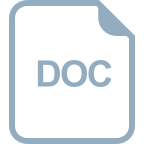




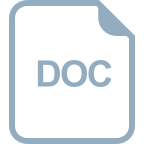
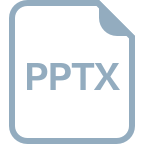
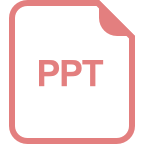
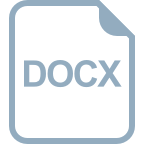
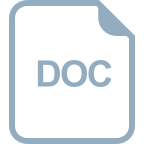
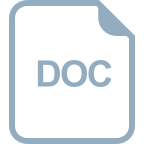
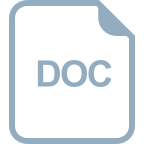
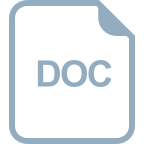

