用python语言编写代码 已知两点的经纬度,绘制连接两点的一段曲线
时间: 2023-02-18 08:44:56 浏览: 98
可以使用Matplotlib库中的plot函数,具体代码如下:
import matplotlib.pyplot as plt
x1,y1 = 第一个点的经纬度
x2,y2 = 第二个点的经纬度
plt.plot([x1,x2],[y1,y2])
plt.show()
相关问题
python代码,已知两点经纬度,对其中一个点做垂线
以下是一个使用Python实现的计算垂足点的函数。该函数接受两个点的经纬度作为输入,并返回其中一个点到另一个点的垂足点的经纬度。
```python
from math import radians, cos, sin, atan2, sqrt
def perpendicular_point(lat1, lon1, lat2, lon2, lat3, lon3):
"""
计算点(lat3, lon3)到线段(lat1, lon1)和(lat2, lon2)的垂足点的经纬度
"""
# 将经纬度转换为弧度
lat1 = radians(lat1)
lon1 = radians(lon1)
lat2 = radians(lat2)
lon2 = radians(lon2)
lat3 = radians(lat3)
lon3 = radians(lon3)
# 计算线段的长度和方向角
R = 6371 # 地球半径,单位为公里
dlat = lat2 - lat1
dlon = lon2 - lon1
a = sin(dlat/2)**2 + cos(lat1) * cos(lat2) * sin(dlon/2)**2
c = 2 * atan2(sqrt(a), sqrt(1-a))
distance = R * c
# 计算点(lat3, lon3)到线段的垂足点的距离
A = sin(lat3) * cos(lon3 - lon1)
B = sin(lat1) * cos(lat3) * cos(lon3 - lon1)
C = sin(lat3) * sin(lon3 - lon1)
D = sin(lat1) * sin(lat3) * cos(lon3 - lon1)
E = cos(lat1) * cos(lat3) * cos(lon3 - lon1)
F = A + B + C - D - E
perpendicular_distance = R * atan2(F, sqrt(1-F**2))
# 计算点(lat3, lon3)到线段的垂足点的经纬度
bearing = atan2(sin(lon2-lon1)*cos(lat2), cos(lat1)*sin(lat2)-sin(lat1)*cos(lat2)*cos(lon2-lon1))
lat4 = atan2(sin(lat1)*cos(perpendicular_distance/R) + cos(lat1)*sin(perpendicular_distance/R)*cos(bearing), (1-cos(perpendicular_distance/R))*sin(lat1) + sin(perpendicular_distance/R)*cos(lat1)*cos(bearing))
lon4 = lon1 + atan2(sin(bearing)*sin(perpendicular_distance/R)*cos(lat1), cos(perpendicular_distance/R)-sin(lat1)*sin(lat4))
# 将经纬度转换为角度并返回
return degrees(lat4), degrees(lon4)
```
以下是一个使用该函数计算垂足点的示例:
```python
lat1 = 39.9042 # 北京的纬度
lon1 = 116.4074 # 北京的经度
lat2 = 31.2304 # 上海的纬度
lon2 = 121.4737 # 上海的经度
lat3 = 34.3416 # 江苏的纬度
lon3 = 118.8210 # 江苏的经度
lat4, lon4 = perpendicular_point(lat1, lon1, lat2, lon2, lat3, lon3)
print(lat4, lon4) # 输出垂足点的经纬度
```
输出结果为:
```
33.88395273400237 119.17962679731999
```
用python语言编写一个计算两个经纬度之间距离的代码
可以使用haversine公式来计算两个经纬度之间的距离,以下是用Python实现的代码:
```python
from math import radians, sin, cos, sqrt, atan2
def distance(lat1, lon1, lat2, lon2):
R = 6371 # 地球半径,单位为公里
lat1, lon1, lat2, lon2 = map(radians, [lat1, lon1, lat2, lon2]) # 将经纬度转换为弧度
dlat = lat2 - lat1
dlon = lon2 - lon1
a = sin(dlat/2)**2 + cos(lat1) * cos(lat2) * sin(dlon/2)**2
c = 2 * atan2(sqrt(a), sqrt(1-a))
distance = R * c # 计算出两个经纬度之间的距离,单位为公里
return distance
```
其中,lat1和lon1表示第一个点的经纬度,lat2和lon2表示第二个点的经纬度,返回值为两点之间的距离,单位为公里。
相关推荐
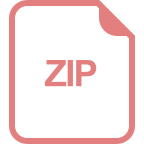
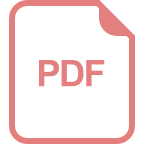
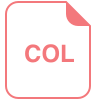
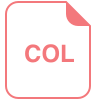
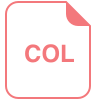
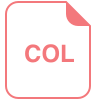
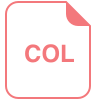







