纹理矩阵 转bitmap
时间: 2023-08-08 11:10:15 浏览: 145
要将纹理矩阵转换为Bitmap,可以使用OpenGL ES将纹理渲染到一个Framebuffer对象中,并使用glReadPixels()方法将像素数据读回到一个ByteBuffer中。然后,可以使用BitmapFactory的decodeByteArray()方法将ByteBuffer中的像素数据解码为Bitmap。
以下是将纹理矩阵转换为Bitmap的代码示例:
```java
// 创建一个Framebuffer对象
int[] framebuffers = new int[1];
GLES20.glGenFramebuffers(1, framebuffers, 0);
int framebufferId = framebuffers[0];
GLES20.glBindFramebuffer(GLES20.GL_FRAMEBUFFER, framebufferId);
// 创建一个纹理并将其附加到Framebuffer对象上
int[] textures = new int[1];
GLES20.glGenTextures(1, textures, 0);
int textureId = textures[0];
GLES20.glBindTexture(GLES20.GL_TEXTURE_2D, textureId);
GLES20.glTexImage2D(GLES20.GL_TEXTURE_2D, 0, GLES20.GL_RGBA, width, height, 0, GLES20.GL_RGBA, GLES20.GL_UNSIGNED_BYTE, null);
GLES20.glFramebufferTexture2D(GLES20.GL_FRAMEBUFFER, GLES20.GL_COLOR_ATTACHMENT0, GLES20.GL_TEXTURE_2D, textureId, 0);
// 检查Framebuffer是否完整
int status = GLES20.glCheckFramebufferStatus(GLES20.GL_FRAMEBUFFER);
if (status != GLES20.GL_FRAMEBUFFER_COMPLETE) {
throw new RuntimeException("Framebuffer is not complete");
}
// 绑定Framebuffer并渲染纹理
GLES20.glViewport(0, 0, width, height);
GLES20.glBindFramebuffer(GLES20.GL_FRAMEBUFFER, framebufferId);
GLES20.glUseProgram(program);
GLES20.glVertexAttribPointer(positionHandle, 2, GLES20.GL_FLOAT, false, 0, vertexBuffer);
GLES20.glVertexAttribPointer(texCoordHandle, 2, GLES20.GL_FLOAT, false, 0, texCoordBuffer);
GLES20.glUniformMatrix4fv(mtxHandle, 1, false, mtx, 0);
GLES20.glActiveTexture(GLES20.GL_TEXTURE0);
GLES20.glBindTexture(GLES11Ext.GL_TEXTURE_EXTERNAL_OES, textureId);
GLES20.glUniform1i(texSamplerHandle, 0);
GLES20.glDrawArrays(GLES20.GL_TRIANGLE_STRIP, 0, 4);
// 读取像素数据并创建一个Bitmap
ByteBuffer buffer = ByteBuffer.allocateDirect(width * height * 4);
buffer.order(ByteOrder.nativeOrder());
GLES20.glReadPixels(0, 0, width, height, GLES20.GL_RGBA, GLES20.GL_UNSIGNED_BYTE, buffer);
Bitmap bitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
bitmap.copyPixelsFromBuffer(buffer);
// 解绑纹理和Framebuffer并删除它们
GLES20.glBindTexture(GLES20.GL_TEXTURE_2D, 0);
GLES20.glDeleteTextures(1, textures, 0);
GLES20.glBindFramebuffer(GLES20.GL_FRAMEBUFFER, 0);
GLES20.glDeleteFramebuffers(1, framebuffers, 0);
```
需要注意的是,这个代码示例假设当前上下文中存在一个有效的OpenGL ES程序,并且已经获得了程序中各个属性和uniform变量的句柄。如果您需要更详细的代码示例,请告诉我您正在使用的平台和开发环境。
阅读全文
相关推荐
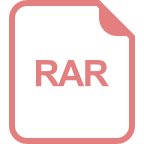
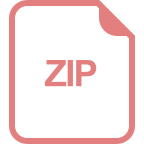
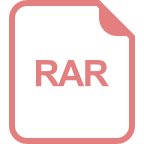
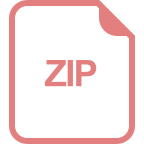
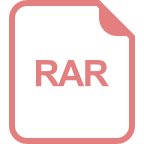
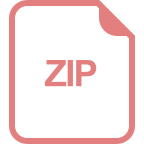
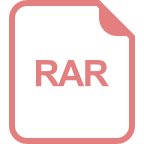
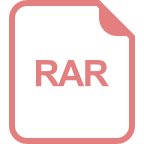
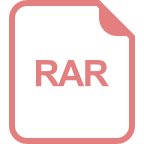
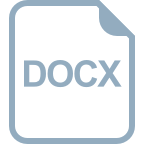
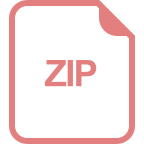
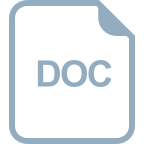
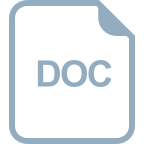
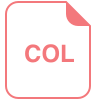


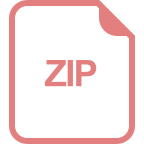
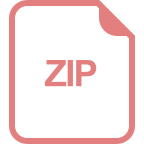
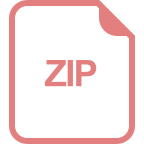