QT 如何用多线程实现数据处理和界面显示刷新速度够快
时间: 2023-07-16 12:17:03 浏览: 175
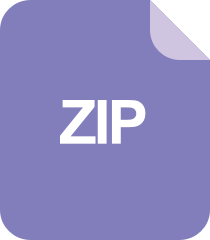
qt实现多线程刷新界面.zip

在QT中,可以使用QThread类来实现多线程。具体步骤如下:
1. 创建一个继承自QThread的子类,重写其run()函数,该函数中实现数据处理的逻辑。
2. 在主界面中创建一个定时器,定时刷新界面。
3. 将处理数据的子线程对象移动到子线程中运行,启动线程。
4. 在定时器中获取处理结果,更新界面。
示例代码如下:
```cpp
// 数据处理线程
class DataProcessThread : public QThread
{
Q_OBJECT
public:
void run() override
{
// 处理数据
}
signals:
void resultReady(const QString& result); // 处理结果信号
};
// 主界面类
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
private slots:
void updateUI(); // 定时器槽函数
private:
QTimer* m_timer; // 定时器
QLabel* m_label; // 显示数据的控件
DataProcessThread* m_thread; // 数据处理线程
};
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
// 创建控件
m_label = new QLabel(this);
// 创建定时器
m_timer = new QTimer(this);
connect(m_timer, &QTimer::timeout, this, &MainWindow::updateUI);
// 创建线程
m_thread = new DataProcessThread(this);
connect(m_thread, &DataProcessThread::resultReady, this, [this](const QString& result) {
m_label->setText(result);
});
// 启动线程
m_thread->start();
// 启动定时器
m_timer->start(100); // 100ms刷新一次界面
}
void MainWindow::updateUI()
{
// 更新界面
}
```
需要注意的是,需要使用信号槽机制将处理结果传递给主线程更新界面。另外,需要保证处理数据的线程不会阻塞主线程,否则会导致界面卡顿。
阅读全文
相关推荐
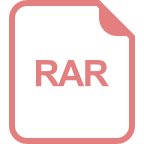
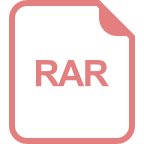
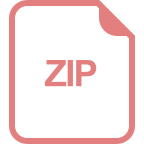
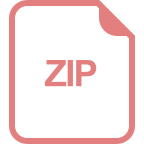
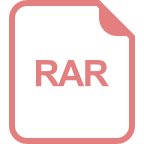
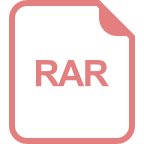
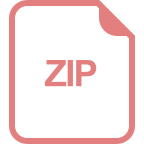
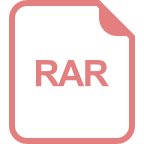
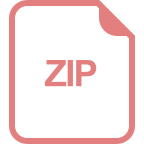
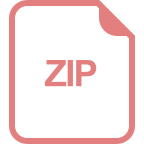
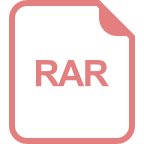
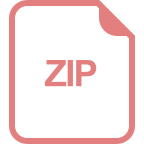
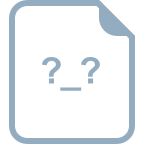
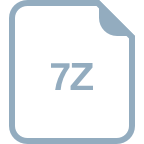