建立顺序栈,实现入栈、出栈、获取栈顶元素操作,并利用顺序栈实现表达式的求解
时间: 2024-05-09 16:15:23 浏览: 10
好的,下面是建立顺序栈的代码实现,其中使用了模板类:
```C++
template <typename T>
class SeqStack {
private:
T* data; // 存储栈元素的数组
int top; // 栈顶指针
int maxSize; // 栈的最大容量
public:
SeqStack(int size = 10); // 构造函数
~SeqStack(); // 析构函数
bool push(T x); // 入栈
bool pop(); // 出栈
T getTop(); // 获取栈顶元素
bool isEmpty(); // 判断栈是否为空
bool isFull(); // 判断栈是否已满
};
template <typename T>
SeqStack<T>::SeqStack(int size) {
data = new T[size];
top = -1;
maxSize = size;
}
template <typename T>
SeqStack<T>::~SeqStack() {
delete[] data;
}
template <typename T>
bool SeqStack<T>::push(T x) {
if (isFull()) {
return false;
}
data[++top] = x;
return true;
}
template <typename T>
bool SeqStack<T>::pop() {
if (isEmpty()) {
return false;
}
top--;
return true;
}
template <typename T>
T SeqStack<T>::getTop() {
if (isEmpty()) {
return NULL;
}
return data[top];
}
template <typename T>
bool SeqStack<T>::isEmpty() {
return top == -1;
}
template <typename T>
bool SeqStack<T>::isFull() {
return top == maxSize - 1;
}
```
接下来,我们利用顺序栈实现表达式的求解。具体步骤如下:
1. 将中缀表达式转换为后缀表达式;
2. 从左至右扫描后缀表达式;
3. 如果是操作数,直接压入栈;
4. 如果是运算符,弹出栈顶的两个操作数进行运算,并将结果压回栈中。
以下是代码实现:
```C++
int evaluate(string s) {
SeqStack<int> nums(100);
for (int i = 0; i < s.length(); i++) {
if (isdigit(s[i])) {
nums.push(s[i] - '0');
} else {
int b = nums.getTop(); nums.pop();
int a = nums.getTop(); nums.pop();
switch (s[i]) {
case '+': nums.push(a + b); break;
case '-': nums.push(a - b); break;
case '*': nums.push(a * b); break;
case '/': nums.push(a / b); break;
}
}
}
return nums.getTop();
}
string infix2postfix(string s) {
SeqStack<char> ops(100);
string postfix = "";
for (int i = 0; i < s.length(); i++) {
if (isdigit(s[i])) {
postfix += s[i];
} else if (s[i] == '(') {
ops.push(s[i]);
} else if (s[i] == ')') {
while (ops.getTop() != '(') {
postfix += ops.getTop();
ops.pop();
}
ops.pop();
} else {
while (!ops.isEmpty() && priority(ops.getTop()) >= priority(s[i])) {
postfix += ops.getTop();
ops.pop();
}
ops.push(s[i]);
}
}
while (!ops.isEmpty()) {
postfix += ops.getTop();
ops.pop();
}
return postfix;
}
```
其中,`infix2postfix` 函数用于将中缀表达式转换为后缀表达式,`evaluate` 函数用于求出表达式的结果。
这里给出一个测试用例:
```C++
string infix = "3*(2+4)-1";
string postfix = infix2postfix(infix);
cout << postfix << endl; // 324+*
cout << evaluate(postfix) << endl; // 15
```
以上就是建立顺序栈,实现入栈、出栈、获取栈顶元素操作,并利用顺序栈实现表达式的求解的代码实现。
相关推荐
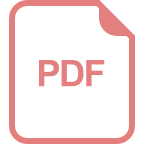
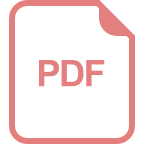














