numpy转tensor
时间: 2023-10-19 13:03:52 浏览: 92
可以使用PyTorch中的`torch.tensor()`函数将NumPy数组转换为PyTorch张量。以下是一个示例代码:
```python
import numpy as np
import torch
# 创建一个NumPy数组
arr = np.array([[1, 2], [3, 4]])
# 将NumPy数组转换为PyTorch张量
tensor = torch.tensor(arr)
print(tensor)
```
输出:
```
tensor([[1, 2],
[3, 4]], dtype=torch.int32)
```
注意,`torch.tensor()`函数会将输入数据的类型转换为默认的PyTorch张量数据类型。如果需要指定数据类型,可以使用`dtype`参数,例如:
```python
# 将NumPy数组转换为FloatTensor类型的PyTorch张量
tensor = torch.tensor(arr, dtype=torch.float32)
```
相关问题
numpy转换tensor
可以使用torch.tensor()或者torch.from_numpy()函数将numpy数组转换为torch中的tensor数据类型。下面是一个例子:
import numpy as np
import torch
arr = np.array([1, 2, 3])
tensor = torch.tensor(arr)
print(tensor)
输出结果为:
tensor([1, 2, 3])
需要注意的是,torch.tensor()会创建一个新的tensor,而torch.from_numpy()则是将numpy数组转换为tensor并且共享内存空间,修改其中一个也会影响到另一个。
numpy 转tensor
要使用 TensorFlow 中的张量,需要将 numpy 数组转换为张量。可以使用 `tf.convert_to_tensor` 函数将 numpy 数组转换为张量。例如:
```python
import numpy as np
import tensorflow as tf
# 创建一个 numpy 数组
arr = np.array([[1, 2], [3, 4]])
# 将 numpy 数组转换为 TensorFlow 张量
tensor = tf.convert_to_tensor(arr)
print(tensor)
```
输出结果为:
```
tf.Tensor(
[[1 2]
[3 4]], shape=(2, 2), dtype=int64)
```
在转换时,可以通过 `dtype` 参数指定所需的数据类型。例如,将 numpy 数组转换为浮点数张量:
```python
# 将 numpy 数组转换为浮点数张量
tensor = tf.convert_to_tensor(arr, dtype=tf.float32)
print(tensor)
```
输出结果为:
```
tf.Tensor(
[[1. 2.]
[3. 4.]], shape=(2, 2), dtype=float32)
```
阅读全文
相关推荐
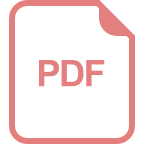
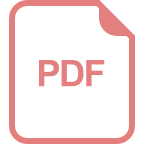
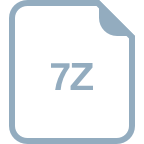


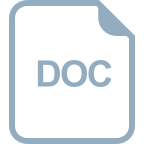









