funtion_label: printf("*****************Funtion Test*****************\n"); unsigned char pPwd[4]={0}; unsigned char UserContent[32]; nRet = ZAZReadIndexTable(nhanle,DEV_ADDR,0,UserContent); if(nRet != ZAZ_OK) printf("1. ZAZReadIndexTable is Fail ,errorr code = %d......\n",nRet); else { printf("1. ZAZReadIndexTable ok \n"); printarrbit(UserContent,32); }
时间: 2023-08-25 18:05:33 浏览: 69
根据代码,这是一个函数的实现,其中包含以下步骤:
1. 打印“Funtion Test”的提示信息;
2. 初始化一个长度为4的unsigned char类型数组pPwd,并将其所有元素都赋值为0;
3. 初始化一个长度为32的unsigned char类型数组UserContent;
4. 调用ZAZReadIndexTable函数读取数据,其中包含4个参数:nhanle表示句柄,DEV_ADDR表示设备地址,0表示索引号,UserContent表示读取到的数据;
5. 判断读取数据的返回值,如果不等于ZAZ_OK,则打印“ZAZReadIndexTable is Fail ,errorr code = %d......\n”的提示信息,并将返回值打印出来;
6. 如果读取数据的返回值等于ZAZ_OK,则打印“ZAZReadIndexTable ok”提示信息,并调用printarrbit函数打印UserContent数组中每个元素的二进制位。
相关问题
As a second example, the goal is to write inline assembler code, that takes a string as input, and calculates the length of the string. Recall that strings in C are represented as arrays of char and each char has a size of 1 byte. Make sure to use the correct assembler-load instruction to get the next character. Strings are terminated by the \0 (the value 0, not the symbol 0), which doesn’t count as part of the string. To test the result, you can compare it with the result of the libc function strlen or with the C function presented in an earlier class.
Here's an example of inline assembler code that calculates the length of a string:
```c
#include <stdio.h>
int main() {
char str[] = "Hello, world!";
int len;
asm volatile (
"movl $0, %0\n\t" // initialize counter to 0
"1:\n\t"
"movb (%1), %%al\n\t" // load next character into al
"cmpb $0, %%al\n\t" // compare with null terminator
"je 2f\n\t" // if equal, jump to end
"incl %0\n\t" // increment counter
"incl %1\n\t" // increment pointer
"jmp 1b\n\t" // jump to beginning of loop
"2:\n\t"
: "=r" (len) // output: len = counter
: "r" (str) // input: str = pointer to string
: "%eax" // clobbered register: eax
);
printf("Length of string: %d\n", len);
return 0;
}
```
Explanation:
- The `asm volatile` statement tells the compiler that this is inline assembler code that may modify registers and memory, and that it should not optimize or reorder instructions.
- The `movl $0, %0` initializes the counter to 0 and assigns it to the output variable `len`.
- The label `1:` marks the beginning of a loop that processes each character of the string.
- The `movb (%1), %%al` instruction loads the next character into the `al` register.
- The `cmpb $0, %%al` instruction compares the character with the null terminator.
- The `je 2f` instruction jumps to the label `2:` if the character is a null terminator.
- The `incl %0` instruction increments the counter.
- The `incl %1` instruction increments the pointer to the next character.
- The `jmp 1b` instruction jumps back to the beginning of the loop.
- The label `2:` marks the end of the loop.
- The output operand `=r` (len) tells the compiler that `len` is an output variable that will be stored in a register.
- The input operand `"r" (str)` tells the compiler that `str` is an input variable that will be stored in a register.
- The clobbered register `%eax` tells the compiler that the `eax` register may be modified by the inline assembler code.
lightgbm3分类算法,输入439*800,439个样本,每个样本有800个特征,输出为439*3,每个样本用3位编码代表样本类别,matlab代码
LightM是一种高效的梯度提升框架,它提供了Python、R、C++等多种语言的接口。如果您想要在Matlab中实现LightGBM算法,可以使用LightGBM官方提供的C++接口,然后在Matlab中调用C++接口。以下是可能的实现步骤:
1. 安装LightGBM C++库
请参考LightGBM官方文档,下载并安装对应的C++库。
2. 编写C++代码
在C++中编写LightGBM分类算法的代码,并将其封装为一个可调用的函数。以下是一个示例代码:
```cpp
#include <iostream>
#include <cstdio>
#include <LightGBM/c_api.h>
int main(int argc, char** argv) {
// Load data
std::string train_file = "train_data.txt";
std::string test_file = "test_data.txt";
std::string model_file = "model.txt";
std::string param_str = "task=train num_class=3";
const char* data_filename = train_file.c_str();
const char* test_filename = test_file.c_str();
const char* model_filename = model_file.c_str();
const char* param_filename = param_str.c_str();
int num_threads = 0;
int early_stopping_round = 10;
double learning_rate = 0.1;
int num_iterations = 1000;
int num_leaves = 31;
// Load training data
int num_train_samples = 439;
int num_features = 800;
double* data = new double[num_train_samples*num_features];
double* label = new double[num_train_samples];
// Load data from file
// ...
// Create dataset
const char* data_format = "array";
LGBM_DatasetHandle train_data;
LGBM_DatasetCreateFromMat(data, data_format, num_train_samples, num_features, 1, label, -1, NULL, &train_data);
// Set parameters
LGBM_ParamsHandle params;
LGBM_CreateParams(¶ms);
LGBM_SetParam(params, "learning_rate", std::to_string(learning_rate).c_str());
LGBM_SetParam(params, "num_iterations", std::to_string(num_iterations).c_str());
LGBM_SetParam(params, "num_leaves", std::to_string(num_leaves).c_str());
// Train model
LGBM_BoosterHandle booster;
LGBM_BoosterCreate(train_data, param_filename, &booster);
for (int i = 0; i < num_iterations; ++i) {
LGBM_BoosterUpdateOneIter(booster);
if (LGBM_BoosterGetCurrentIteration(booster) % 10 == 0) {
int is_finished;
LGBM_BoosterIsFinished(booster, &is_finished);
if (is_finished) {
break;
}
double score;
LGBM_BoosterEvalOneMetric(booster, "multi_logloss", &score);
std::printf("Iteration %d, multi_logloss = %g\n", LGBM_BoosterGetCurrentIteration(booster), score);
}
}
// Save model
LGBM_BoosterSaveModel(booster, -1, -1, model_filename);
// Load test data
int num_test_samples = 100;
double* test_data = new double[num_test_samples*num_features];
double* test_label = new double[num_test_samples];
// Load data from file
// ...
// Create dataset
LGBM_DatasetHandle test_data;
LGBM_DatasetCreateFromMat(test_data, data_format, num_test_samples, num_features, 1, test_label, -1, NULL, &test_data);
// Predict
LGBM_BoosterPredictForMat(booster, test_data, num_test_samples, num_features, 1, LGBM_PREDICT_NORMAL, -1, NULL, NULL);
// Free memory
delete[] data;
delete[] label;
delete[] test_data;
delete[] test_label;
LGBM_DatasetFree(train_data);
LGBM_DatasetFree(test_data);
LGBM_ParamsFree(params);
LGBM_BoosterFree(booster);
return 0;
}
```
3. 编写Matlab代码
在Matlab中编写调用上述C++函数的代码。以下是一个示例代码:
```matlab
% Load data
data = dlmread('train_data.txt');
label = dlmread('train_label.txt');
% Call C++ function
results = call_lightgbm(data, label);
% Save results
dlmwrite('results.txt', results);
```
在上述示例代码中,`call_lightgbm`函数是调用C++函数的Matlab函数,它可以使用Matlab的MEX机制实现。具体实现方式请参考Matlab官方文档。
希望这些信息能够对您有所帮助!
阅读全文
相关推荐
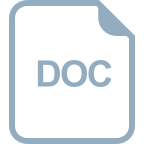
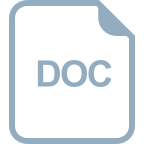
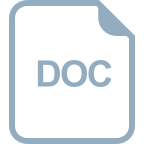
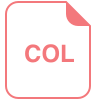
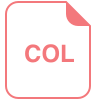
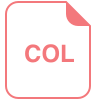
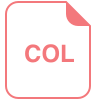

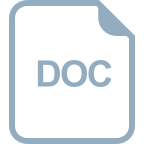
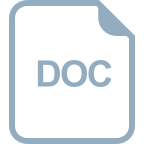
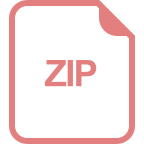
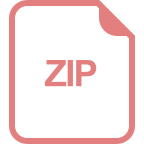
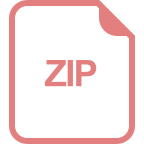
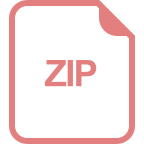
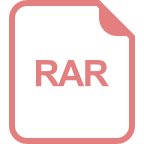
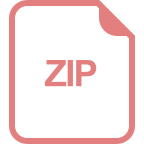
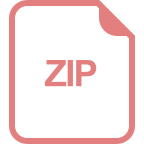