c语言作业迷宫代码,用c语言实现迷宫求解完美源代码
时间: 2024-01-21 08:19:18 浏览: 27
以下是用C语言实现迷宫求解的完美源代码:
```c
#include <stdio.h>
#define MAX_ROW 5
#define MAX_COL 5
int map[MAX_ROW][MAX_COL] = {
{0, 1, 0, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 0, 0, 1, 0},
};
struct point {
int row, col, parent;
} queue[512];
int head = 0, tail = 0;
void enqueue(struct point p)
{
queue[tail++] = p;
}
struct point dequeue(void)
{
return queue[head++];
}
int is_empty(void)
{
return head == tail;
}
int main(void)
{
struct point p = {0, 0, -1};
map[p.row][p.col] = 2;
enqueue(p);
while (!is_empty()) {
struct point cur = dequeue();
if (cur.row == MAX_ROW - 1 && cur.col == MAX_COL - 1) {
printf("(%d, %d)\n", cur.row, cur.col);
while (cur.parent != -1) {
cur = queue[cur.parent];
printf("(%d, %d)\n", cur.row, cur.col);
}
return 0;
}
if (cur.col+1 < MAX_COL && map[cur.row][cur.col+1] == 0) { /* right */
struct point p = {cur.row, cur.col+1, head-1};
enqueue(p);
map[p.row][p.col] = 2;
}
if (cur.row+1 < MAX_ROW && map[cur.row+1][cur.col] == 0) { /* down */
struct point p = {cur.row+1, cur.col, head-1};
enqueue(p);
map[p.row][p.col] = 2;
}
if (cur.col-1 >= 0 && map[cur.row][cur.col-1] == 0) { /* left */
struct point p = {cur.row, cur.col-1, head-1};
enqueue(p);
map[p.row][p.col] = 2;
}
if (cur.row-1 >= 0 && map[cur.row-1][cur.col] == 0) { /* up */
struct point p = {cur.row-1, cur.col, head-1};
enqueue(p);
map[p.row][p.col] = 2;
}
}
printf("No path found!\n");
return 0;
}
```
这个代码使用广度优先搜索算法(BFS)来解决迷宫问题。它使用一个队列来存储所有已经被访问的点,然后根据当前点的位置,将它周围没有被访问过的点加入队列中,并标记为已访问。这个过程一直持续到找到终点或者队列为空。如果找到了终点,我们就可以从终点开始倒推,直到回到起点,得到完整路径。
这个代码中的 `map` 数组表示迷宫的地图,0 表示可以通过的路,1 表示墙,2 表示已经访问过的点。`struct point` 结构体表示一个点的位置和它的父节点在队列中的位置。`enqueue` 和 `dequeue` 函数用来操作队列。`is_empty` 函数用来检查队列是否为空。在主函数中,我们从起点开始,将它加入队列中,并标记为已访问。然后开始搜索,直到找到终点或者队列为空。如果找到了终点,我们就可以倒推路径并输出结果。如果没有找到终点,就输出“无解”。
相关推荐
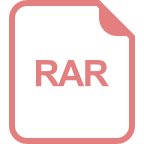
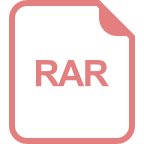














