c语言用栈实现迷宫的生成及迷宫的求解的源代码
时间: 2024-10-08 20:26:45 浏览: 85
在C语言中,使用栈来实现迷宫的生成和求解是一种常见的算法设计思路,其中广度优先搜索(BFS)常用于迷宫生成,而回溯法或类似算法可以用于解决路径寻找的问题。这里是一个简化版的迷宫生成和求解的伪代码示例:
```c
// 定义迷宫的结构体
typedef struct {
int maze[ROW][COL]; // 迷宫矩阵,0表示空地,1表示墙
int visited[ROW][COL]; // 记录已访问位置
} Maze;
// 创建一个新迷宫
void create_maze(Maze *maze) {
stack_t cellStack; // 使用栈存储待探索的单元格
// 初始化起点和填充边界
init_stack(&cellStack, maze->start_row, maze->start_col);
maze->maze[maze->start_row][maze->start_col] = 0;
maze->visited[maze->start_row][maze->start_col] = 1;
while (!is_empty(&cellStack)) { // 当栈非空时
int row, col;
pop_stack(&cellStack, &row, &col); // 取出顶部单元格
// 检查上下左右四个方向
if (check_neighbors(maze, row, col)) {
// 如果相邻位置未访问过,标记并加入栈
mark_neighbor(maze, row, col);
push_stack(&cellStack, row + 1, col); // 上方
push_stack(&cellStack, row - 1, col); // 下方
push_stack(&cellStack, row, col + 1); // 右侧
push_stack(&cellStack, row, col - 1); // 左侧
}
}
}
// 解决迷宫问题,从起点到终点
int solve_maze(Maze *maze, int start_row, int start_col, int end_row, int end_col) {
stack_t pathStack; // 路径追踪栈
maze->maze[start_row][start_col] = 0; // 标记起点
push_stack(&pathStack, start_row, start_col);
while (!is_empty(&pathStack)) {
int current_row, current_col;
pop_stack(&pathStack, ¤t_row, ¤t_col);
if (current_row == end_row && current_col == end_col) {
return 1; // 找到了路径
}
// 检查上、下、左三个邻居是否为目标或已走过的路径
if (can_move(maze, current_row, current_col, end_row, end_col)) {
mark_path(maze, current_row, current_col);
push_stack(&pathStack, current_row + 1, current_col); // 上方
push_stack(&pathStack, current_row - 1, current_col); // 下方
push_stack(&pathStack, current_row, current_col - 1); // 左侧
}
}
return 0; // 没有找到路径
}
阅读全文
相关推荐
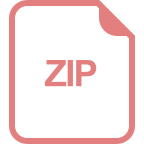
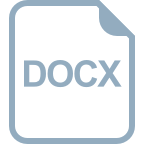
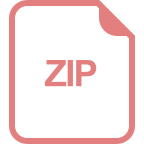
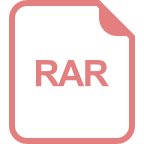
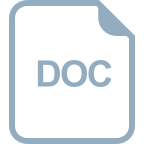
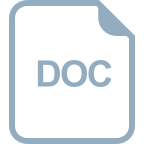
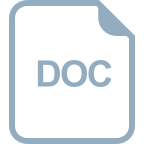
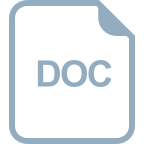
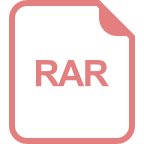
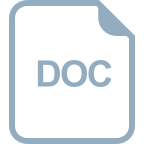
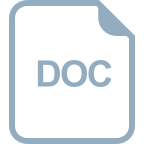
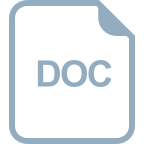
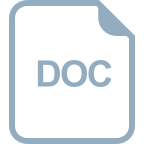
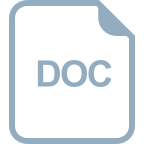
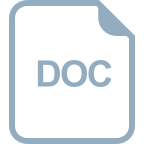

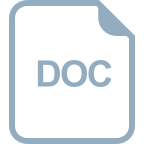
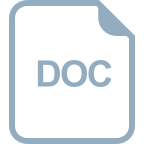
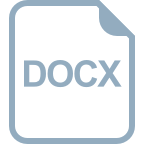