以链表为集合将运算符重载实现交并集在不使用STL库的情况下
时间: 2023-05-30 20:02:20 浏览: 59
以下是使用链表实现交并集的代码:
```cpp
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
Node(int d) {
data = d;
next = NULL;
}
};
class LinkedList {
private:
Node* head;
public:
LinkedList() {
head = NULL;
}
void insert(int data) {
Node* newNode = new Node(data);
if (head == NULL) {
head = newNode;
}
else {
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
void print() {
Node* temp = head;
while (temp != NULL) {
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
}
LinkedList intersection(LinkedList list) {
LinkedList result;
Node* temp = head;
while (temp != NULL) {
if (list.contains(temp->data)) {
result.insert(temp->data);
}
temp = temp->next;
}
return result;
}
LinkedList unionSet(LinkedList list) {
LinkedList result = list;
Node* temp = head;
while (temp != NULL) {
if (!result.contains(temp->data)) {
result.insert(temp->data);
}
temp = temp->next;
}
return result;
}
bool contains(int data) {
Node* temp = head;
while (temp != NULL) {
if (temp->data == data) {
return true;
}
temp = temp->next;
}
return false;
}
};
int main() {
LinkedList list1;
list1.insert(1);
list1.insert(2);
list1.insert(3);
list1.insert(4);
LinkedList list2;
list2.insert(3);
list2.insert(4);
list2.insert(5);
list2.insert(6);
LinkedList intersection = list1.intersection(list2);
LinkedList unionSet = list1.unionSet(list2);
cout << "Intersection: ";
intersection.print();
cout << "Union Set: ";
unionSet.print();
return 0;
}
```
在上面的代码中,我们首先定义了一个Node类和LinkedList类。Node类表示链表中的节点,LinkedList类表示链表。
LinkedList类包含以下方法:
- insert(int data):向链表中插入一个节点
- print():打印链表中的所有节点
- intersection(LinkedList list):返回当前链表和另一个链表的交集
- unionSet(LinkedList list):返回当前链表和另一个链表的并集
- contains(int data):检查链表中是否包含给定的数据
在main函数中,我们创建了两个链表list1和list2,然后调用intersection和unionSet方法来计算它们的交集和并集,并打印结果。
需要注意的是,这个实现并没有使用STL库,而是使用了自定义的链表类。
相关推荐
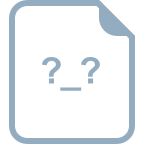














