java文件复制方法_Java复制文件–用Java复制文件的4种方法
时间: 2024-03-16 09:47:07 浏览: 55
好的,以下是四种Java复制文件的方法:
1.使用Java IO类库中的FileInputStream和FileOutputStream类来复制文件。这是一种基本的方法,可以用于小文件的复制。示例代码如下:
```
public static void copyFileUsingStream(File source, File dest) throws IOException {
InputStream is = null;
OutputStream os = null;
try {
is = new FileInputStream(source);
os = new FileOutputStream(dest);
byte[] buffer = new byte[1024];
int length;
while ((length = is.read(buffer)) > 0) {
os.write(buffer, 0, length);
}
} finally {
is.close();
os.close();
}
}
```
2.使用Java NIO类库中的Files类来复制文件。这种方法可以处理大文件,但是需要Java 7或以上版本。示例代码如下:
```
public static void copyFileUsingJava7Files(File source, File dest) throws IOException {
Files.copy(source.toPath(), dest.toPath());
}
```
3.使用Apache Commons IO类库中的FileUtils类来复制文件。这种方法可以处理大文件,并且不需要手动关闭流。示例代码如下:
```
public static void copyFileUsingApacheCommonsIO(File source, File dest) throws IOException {
FileUtils.copyFile(source, dest);
}
```
4.使用Java IO类库中的BufferedInputStream和BufferedOutputStream类来复制文件。这种方法可以处理大文件,并且不需要Java 7或以上版本。示例代码如下:
```
public static void copyFileUsingBufferedStream(File source, File dest) throws IOException {
InputStream is = null;
OutputStream os = null;
try {
is = new BufferedInputStream(new FileInputStream(source));
os = new BufferedOutputStream(new FileOutputStream(dest));
byte[] buffer = new byte[1024];
int length;
while ((length = is.read(buffer)) > 0) {
os.write(buffer, 0, length);
}
} finally {
is.close();
os.close();
}
}
```
希望这些代码对你有所帮助!
阅读全文
相关推荐
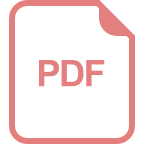
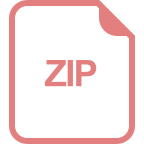
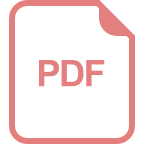














