手写一个swiper
时间: 2023-08-25 13:08:15 浏览: 143
好的,下面是一个简单的手写swiper实现:
```html
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
<div class="swiper-slide">Slide 4</div>
<div class="swiper-slide">Slide 5</div>
</div>
</div>
```
```css
.swiper-container {
position: relative;
height: 300px;
overflow: hidden;
}
.swiper-wrapper {
position: absolute;
width: 100%;
height: 100%;
display: flex;
transition: transform 0.3s ease-out;
}
.swiper-slide {
width: 100%;
height: 100%;
flex-shrink: 0;
margin-right: 20px;
}
```
```js
const container = document.querySelector('.swiper-container');
const wrapper = document.querySelector('.swiper-wrapper');
const slides = document.querySelectorAll('.swiper-slide');
const slideWidth = slides[0].offsetWidth + 20; // 计算每个slide的宽度
let currentIndex = 0;
let isMoving = false;
// 移动到指定slide
function moveTo(index) {
if (index < 0 || index > slides.length - 1 || isMoving) return;
isMoving = true;
wrapper.style.transform = `translateX(-${slideWidth * index}px)`;
currentIndex = index;
setTimeout(() => {
isMoving = false;
}, 300);
}
// 绑定滑动事件
let startX, startY;
container.addEventListener('touchstart', (e) => {
startX = e.touches[0].clientX;
startY = e.touches[0].clientY;
});
container.addEventListener('touchmove', (e) => {
const deltaX = e.touches[0].clientX - startX;
const deltaY = e.touches[0].clientY - startY;
if (Math.abs(deltaX) > Math.abs(deltaY)) {
e.preventDefault();
wrapper.style.transform = `translateX(-${slideWidth * currentIndex - deltaX}px)`;
}
});
container.addEventListener('touchend', (e) => {
const deltaX = e.changedTouches[0].clientX - startX;
if (Math.abs(deltaX) > slideWidth / 3) {
moveTo(currentIndex + (deltaX > 0 ? -1 : 1));
} else {
moveTo(currentIndex);
}
});
// 初始化
wrapper.style.width = `${slideWidth * slides.length}px`;
moveTo(0);
```
以上代码实现了一个简单的手写swiper,支持滑动切换和左右箭头切换。需要注意的是,这里的样式和交互仅仅是做一个示例,实际使用中还需要考虑更多的细节问题,比如可配置性、性能优化等。
相关推荐
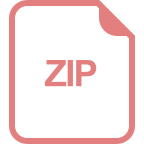
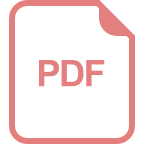

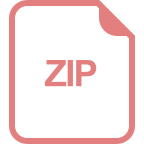
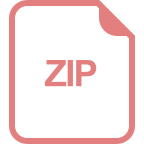
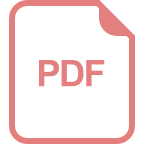
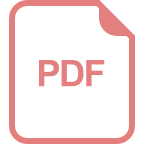
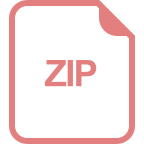
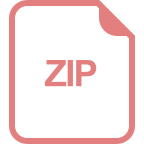
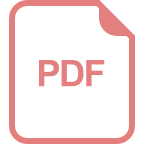
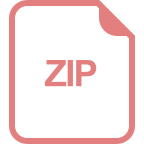
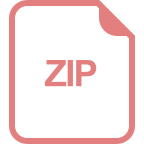



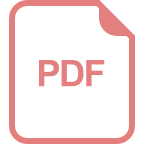