设计一个系统,功能是随机生成1000个1到1000之间的数(浮点型),保留小数点后50位【提示用串表示】,并保存到文件中,并输出最小的10个数。同时,找出这10个数中相差最大的两个数,用数据算法与结构和C语言的知识完成
时间: 2023-10-10 22:14:00 浏览: 44
1. 生成随机数并保存到文件中
可以使用C语言中的rand()函数生成1到1000之间的整数,再将其转换为浮点型,并保留小数点后50位。具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#define MAX_NUM 1000
#define NUM_COUNT 1000
#define DECIMAL_COUNT 50
int main() {
srand((unsigned) time(NULL)); // 初始化随机数种子
char nums[NUM_COUNT][DECIMAL_COUNT+1]; // 存储随机数的数组,+1是为了存储字符串结束符'\0'
int i;
for (i = 0; i < NUM_COUNT; i++) {
int num = rand() % MAX_NUM + 1; // 生成1到1000之间的整数
sprintf(nums[i], "%.50f", (double)num); // 将整数转换为浮点型,并保留小数点后50位
}
// 将随机数保存到文件中
FILE *fp = fopen("random.txt", "w");
if (fp == NULL) {
printf("Failed to open file.\n");
exit(1);
}
for (i = 0; i < NUM_COUNT; i++) {
fprintf(fp, "%s\n", nums[i]); // 将随机数写入文件
}
fclose(fp);
return 0;
}
```
2. 输出最小的10个数
可以使用选择排序对随机数进行排序,然后输出前10个数即可。具体实现如下:
```c
int main() {
// 生成随机数并保存到文件中...
// ...
// 读取文件中的随机数
fp = fopen("random.txt", "r");
if (fp == NULL) {
printf("Failed to open file.\n");
exit(1);
}
double nums[NUM_COUNT];
for (i = 0; i < NUM_COUNT; i++) {
char numStr[DECIMAL_COUNT+1];
fgets(numStr, DECIMAL_COUNT+1, fp); // 从文件中读取一行随机数
nums[i] = atof(numStr); // 将字符串转换为浮点型
}
fclose(fp);
// 选择排序
for (i = 0; i < NUM_COUNT-1; i++) {
int minIdx = i;
for (int j = i+1; j < NUM_COUNT; j++) {
if (nums[j] < nums[minIdx]) {
minIdx = j;
}
}
double temp = nums[i];
nums[i] = nums[minIdx];
nums[minIdx] = temp;
}
// 输出最小的10个数
printf("The smallest 10 numbers are:\n");
for (i = 0; i < 10; i++) {
printf("%.50f\n", nums[i]);
}
return 0;
}
```
3. 找出这10个数中相差最大的两个数
可以先将最小的10个数存储到数组中,然后对该数组进行排序,找出相邻两个数之间差值最大的即可。具体实现如下:
```c
int main() {
// 生成随机数并保存到文件中...
// ...
// 读取文件中的随机数
// ...
// 选择排序
// ...
// 输出最小的10个数
// ...
// 找出这10个数中相差最大的两个数
double smallest10[10];
memcpy(smallest10, nums, sizeof(smallest10)); // 将最小的10个数存储到数组中
for (i = 0; i < 9; i++) { // 对数组进行排序
for (int j = i+1; j < 10; j++) {
if (smallest10[j] < smallest10[i]) {
double temp = smallest10[i];
smallest10[i] = smallest10[j];
smallest10[j] = temp;
}
}
}
double maxDiff = 0.0;
int maxDiffIdx1, maxDiffIdx2;
for (i = 0; i < 9; i++) { // 找出相邻两个数之间差值最大的两个数
double diff = smallest10[i+1] - smallest10[i];
if (diff > maxDiff) {
maxDiff = diff;
maxDiffIdx1 = i;
maxDiffIdx2 = i+1;
}
}
printf("The two numbers with the largest difference are:\n%.50f and %.50f\n", smallest10[maxDiffIdx1], smallest10[maxDiffIdx2]);
return 0;
}
```
相关推荐
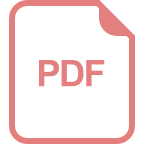















