用结构体编写程序:学生有三个属性:姓名(string型)、年龄(int),成绩(float),编写程序输入三个学生的信息后再将三个学生的信息输出到屏幕上。
时间: 2024-02-07 20:02:33 浏览: 45
好的,这是一个计算机编程问题,可以回答。以下是C++语言编写的程序示例:
```c++
#include <iostream>
using namespace std;
struct Student {
string name;
int age;
float score;
};
int main() {
Student s[3];
for (int i = 0; i < 3; ++i) {
cout << "请输入第" << i+1 << "个学生的信息:" << endl;
cout << "姓名:";
cin >> s[i].name;
cout << "年龄:";
cin >> s[i].age;
cout << "成绩:";
cin >> s[i].score;
}
cout << endl << "三个学生的信息如下:" << endl;
for (int i = 0; i < 3; ++i) {
cout << "姓名:" << s[i].name << "\t";
cout << "年龄:" << s[i].age << "\t";
cout << "成绩:" << s[i].score << endl;
}
return 0;
}
```
运行程序后,依次输入每个学生的姓名、年龄、成绩即可输出三个学生的信息。
相关问题
结构体编写程序:从键盘输入n个学生的6门课程考试成绩,计算每个学生的平均成 绩,并按平均成绩从高到低输出每个学生的信息(包括学号、姓名和6门成绩)。
以下是一个可能的解决方案,使用了结构体和动态内存分配:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct {
char id[20]; // 学号
char name[20]; // 姓名
float scores[6]; // 6门成绩
float avg_score; // 平均成绩
} Student;
int main() {
int n; // 学生个数
printf("请输入学生个数:");
scanf("%d", &n);
// 动态分配内存存储学生数组
Student *students = (Student*)malloc(n * sizeof(Student));
// 输入每个学生的信息
for (int i = 0; i < n; i++) {
printf("请输入第%d个学生的学号、姓名和6门成绩(用空格分隔):", i+1);
scanf("%s %s", students[i].id, students[i].name);
for (int j = 0; j < 6; j++) {
scanf("%f", &students[i].scores[j]);
}
// 计算平均成绩
float sum = 0;
for (int j = 0; j < 6; j++) {
sum += students[i].scores[j];
}
students[i].avg_score = sum / 6.0;
}
// 按平均成绩从高到低排序
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (students[j].avg_score < students[j+1].avg_score) {
// 交换两个学生的信息
Student temp = students[j];
students[j] = students[j+1];
students[j+1] = temp;
}
}
}
// 输出每个学生的信息
for (int i = 0; i < n; i++) {
printf("%s %s ", students[i].id, students[i].name);
for (int j = 0; j < 6; j++) {
printf("%.2f ", students[i].scores[j]);
}
printf("%.2f\n", students[i].avg_score);
}
// 释放动态分配的内存
free(students);
return 0;
}
```
注意,上述代码中使用了 `scanf("%s %s", students[i].id, students[i].name)` 来输入学号和姓名,这里假设学号和姓名中不包含空格。如果学号和姓名中可能包含空格,可以使用 `fgets()` 函数来输入字符串。此外,由于涉及动态内存分配,需要在程序结束前使用 `free()` 函数释放分配的内存。
用C语言中的结构体编写: 输入并显示五个学生的学号、姓名、手机号、语文成绩
和数学成绩。
答案:
在C语言中,可以使用结构体来存储和管理一组相关的数据。在本题中,我们可以定义一个名为“student”的结构体,用于存储学生的信息,包括学号、姓名、手机号、语文成绩和数学成绩。
```c
#include <stdio.h>
#include <string.h>
#define MAX_NAME_LEN 20
#define MAX_PHONE_LEN 20
struct student {
int id;
char name[MAX_NAME_LEN];
char phone[MAX_PHONE_LEN];
float chinese_score;
float math_score;
};
int main() {
int i;
struct student stu[5];
for(i = 0; i < 5; i++) {
printf("Please enter information for student %d:\n", i+1);
printf("ID: ");
scanf("%d", &stu[i].id);
printf("Name: ");
scanf("%s", stu[i].name);
printf("Phone: ");
scanf("%s", stu[i].phone);
printf("Chinese score: ");
scanf("%f", &stu[i].chinese_score);
printf("Math score: ");
scanf("%f", &stu[i].math_score);
printf("\n");
}
printf("ID\tName\tPhone\t\tChinese\tMath\n");
for(i = 0; i < 5; i++) {
printf("%d\t%s\t%s\t", stu[i].id, stu[i].name, stu[i].phone);
printf("%.2f\t%.2f\n", stu[i].chinese_score, stu[i].math_score);
}
return 0;
}
```
在上述代码中,我们首先定义了一个结构体“student”,其中包含五个成员:id、name、phone、chinese_score和math_score。在主函数中,我们创建了一个包含五个“student”结构体的数组,并使用循环依次输入每个学生的信息。最后,我们再次使用循环输出所有学生的信息。
注意,在输入字符串时,需要使用“%s”格式化符,并通过数组名来指定存储字符串的数组。同时,由于字符串数组的大小是有限的,因此需要通过#define指令定义MAX_NAME_LEN和MAX_PHONE_LEN来限制输入的长度,以避免出现内存溢出的问题。
阅读全文
相关推荐
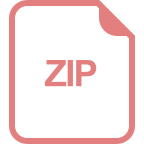
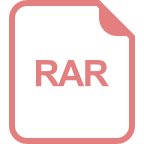













