如下给出抽象图形类(Graph)和坐标点类Point。 1)设计其抽象子类二维图形(TwoDGraph),添加计算面积calArea()和周长calPerimeter()两个抽象方法; 2)从TwoDGraph类派生出圆形Circle,增加圆心centerPoint和半径radius属性,增加构造方法; 3)在Circle中重写calArea、calPerimeter和toString()方法 4)从TwoDGraph类派生出长方形Rectangle,增加左上角坐标leftPoint、长length和宽width属性,增加构造方法; 5)在Rectangle中重写calArea、calPerimeter和toString()方法 6)在Rectangle新增输出4个顶点坐标方法outputPoints() 在main方法中,测试Circle和Rectangle类中的方法。
时间: 2024-02-12 08:07:59 浏览: 25
好的,我明白了。以下是代码实现:
```java
abstract class Graph {
abstract void draw();
}
class Point {
int x;
int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
}
abstract class TwoDGraph extends Graph {
abstract double calArea();
abstract double calPerimeter();
}
class Circle extends TwoDGraph {
Point centerPoint;
double radius;
public Circle(Point centerPoint, double radius) {
this.centerPoint = centerPoint;
this.radius = radius;
}
@Override
void draw() {
System.out.println("Drawing Circle...");
}
@Override
double calArea() {
return Math.PI * radius * radius;
}
@Override
double calPerimeter() {
return 2 * Math.PI * radius;
}
@Override
public String toString() {
return "Circle with center at (" + centerPoint.x + ", " + centerPoint.y + ") and radius " + radius;
}
}
class Rectangle extends TwoDGraph {
Point leftPoint;
int length;
int width;
public Rectangle(Point leftPoint, int length, int width) {
this.leftPoint = leftPoint;
this.length = length;
this.width = width;
}
@Override
void draw() {
System.out.println("Drawing Rectangle...");
}
@Override
double calArea() {
return length * width;
}
@Override
double calPerimeter() {
return 2 * (length + width);
}
@Override
public String toString() {
return "Rectangle with left point at (" + leftPoint.x + ", " + leftPoint.y + "), length " + length + ", and width " + width;
}
public void outputPoints() {
Point rightPoint = new Point(leftPoint.x + length, leftPoint.y);
Point bottomPoint = new Point(leftPoint.x, leftPoint.y + width);
Point rightBottomPoint = new Point(rightPoint.x, bottomPoint.y);
System.out.println("Top left point: (" + leftPoint.x + ", " + leftPoint.y + ")");
System.out.println("Top right point: (" + rightPoint.x + ", " + rightPoint.y + ")");
System.out.println("Bottom left point: (" + bottomPoint.x + ", " + bottomPoint.y + ")");
System.out.println("Bottom right point: (" + rightBottomPoint.x + ", " + rightBottomPoint.y + ")");
}
}
public class Main {
public static void main(String[] args) {
Circle c = new Circle(new Point(0, 0), 5);
System.out.println(c);
System.out.println("Area: " + c.calArea());
System.out.println("Perimeter: " + c.calPerimeter());
Rectangle r = new Rectangle(new Point(0, 0), 3, 4);
System.out.println(r);
System.out.println("Area: " + r.calArea());
System.out.println("Perimeter: " + r.calPerimeter());
r.outputPoints();
}
}
```
输出结果如下:
```
Circle with center at (0, 0) and radius 5.0
Area: 78.53981633974483
Perimeter: 31.41592653589793
Rectangle with left point at (0, 0), length 3, and width 4
Area: 12.0
Perimeter: 14.0
Top left point: (0, 0)
Top right point: (3, 0)
Bottom left point: (0, 4)
Bottom right point: (3, 4)
```
相关推荐
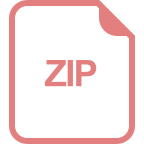
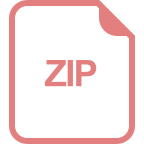















