<template> <table> <thead> <tr> <th>时间段</th> <th v-for="(item, index) in columnCount" :key="index">{{ index + 1 }}</th> </tr> </thead> <tbody> <tr v-for="(item, index) in rowNames" :key="index"> <td>{{ item }}</td> <td v-for="(col, colIndex) in columnCount" :key="colIndex"> <!-- 这里可以填充你要展示的数据 --> </td> </tr> </tbody> </table> </template> <script> export default { data() { return { columnCount: 0, rowNames: [] }; }, mounted() { // 从数据库获取列数和行名 // 假设获取到的数据格式为 { columnCount: 6, rowNames: ['时间段1', '时间段2', ...] } // 这里只是模拟,实际使用时需要替换成真实数据 const data = { columnCount: 6, rowNames: ['时间段1', '时间段2', '时间段3', '时间段4', '时间段5', '时间段6'] }; this.columnCount = data.columnCount; this.rowNames = data.rowNames; } }; </script>解释每一句
时间: 2024-03-03 21:52:57 浏览: 65
好的,我来解释一下这段代码的每一句话的含义:
```
<template>
<table>
<thead>
<tr>
<th>时间段</th>
<th v-for="(item, index) in columnCount" :key="index">{{ index + 1 }}</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in rowNames" :key="index">
<td>{{ item }}</td>
<td v-for="(col, colIndex) in columnCount" :key="colIndex">
<!-- 这里可以填充你要展示的数据 -->
</td>
</tr>
</tbody>
</table>
</template>
```
这段代码定义了一个表格,其中包含了一个表头和一个表身。表头包含了一个“时间段”的标题和一些列,列的数量由 `columnCount` 变量指定。表身包含了若干行,每行对应一个时间段和一些列。
```
<script>
export default {
data() {
return {
columnCount: 0,
rowNames: []
};
},
mounted() {
// 从数据库获取列数和行名
// 假设获取到的数据格式为 { columnCount: 6, rowNames: ['时间段1', '时间段2', ...] }
// 这里只是模拟,实际使用时需要替换成真实数据
const data = { columnCount: 6, rowNames: ['时间段1', '时间段2', '时间段3', '时间段4', '时间段5', '时间段6'] };
this.columnCount = data.columnCount;
this.rowNames = data.rowNames;
}
};
</script>
```
这段代码定义了一个 Vue 组件,并且在组件的 `data` 中定义了两个变量 `columnCount` 和 `rowNames`。`columnCount` 表示列的数量,`rowNames` 表示每一行的名称。在 `mounted` 钩子函数中,我们模拟从数据库获取了列数和行名,并将其存储在 `columnCount` 和 `rowNames` 变量中。
```
<th v-for="(item, index) in columnCount" :key="index">{{ index + 1 }}</th>
```
这行代码使用了 `v-for` 指令,将 `columnCount` 变量中的数量循环渲染出多个 `<th>` 元素,用于展示表格的列。在每个 `<th>` 元素中,我们显示了列的索引(从 1 开始)。
```
<tr v-for="(item, index) in rowNames" :key="index">
<td>{{ item }}</td>
<td v-for="(col, colIndex) in columnCount" :key="colIndex">
<!-- 这里可以填充你要展示的数据 -->
</td>
</tr>
```
这行代码使用了 `v-for` 指令,将 `rowNames` 变量中的每个时间段循环渲染出多个 `<tr>` 元素,用于展示表格的行。在每个 `<tr>` 元素中,我们首先显示该行的时间段名称,然后再使用 `v-for` 指令将该行中的每个列循环渲染出多个 `<td>` 元素,用于填充数据。在每个 `<td>` 元素中,你可以填充你要展示的数据。
`:key` 属性用于优化性能,它告诉 Vue 如何识别每个元素。在这里,我们使用了 `index` 和 `colIndex` 作为元素的 key 值,因为它们是唯一的。
阅读全文
相关推荐
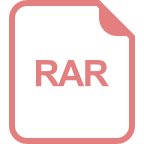

















