基于手工搭建神经网络实现对MNIST手写数字图像识别
时间: 2023-07-29 07:10:40 浏览: 56
好的,这是一个关于实现MNIST手写数字图像识别的问题。首先,我们需要了解MNIST数据集是什么。MNIST数据集是一个手写数字的数据库,由60,000个训练图像和10,000个测试图像组成。每个图像都是28×28像素,灰度图像,表示0到9之间的数字。
为了实现MNIST手写数字图像识别,我们可以使用Python编程语言及其深度学习框架Keras。以下是实现步骤:
1. 导入MNIST数据集
我们可以使用Keras提供的mnist模块来导入MNIST数据集。首先,我们需要安装Keras:`pip install keras`。然后,通过以下代码导入MNIST数据集:
```python
from keras.datasets import mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
```
这里,x_train和x_test是训练集和测试集中的图像数据,y_train和y_test是相应的标签。
2. 数据预处理
我们需要对数据进行预处理,以便在神经网络中使用。首先,我们将图像数据从二维数组(28×28像素)转换为一维数组(784像素)。然后,我们将像素值缩放到0到1之间。
```python
x_train = x_train.reshape(60000, 784)
x_test = x_test.reshape(10000, 784)
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
```
3. 构建神经网络模型
我们可以使用Keras的Sequential模型来构建神经网络模型。我们将使用两个密集层,每个层包含128个神经元,并使用ReLU激活函数。最后,我们在输出层使用softmax激活函数,以获得0到9之间每个数字的预测概率。
```python
from keras.models import Sequential
from keras.layers import Dense
model = Sequential()
model.add(Dense(128, activation='relu', input_shape=(784,)))
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
```
4. 编译和训练模型
我们需要编译模型,并指定损失函数、优化器和评估指标。我们使用交叉熵损失函数、Adam优化器和准确率评估指标。
```python
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
```
然后,我们可以使用训练集对模型进行训练。
```python
model.fit(x_train, y_train, batch_size=128, epochs=10, validation_data=(x_test, y_test))
```
5. 评估模型
最后,我们可以使用测试集评估模型的性能。
```python
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
```
这将输出模型在测试集上的损失和准确率。
希望这个回答对你有所帮助!
相关推荐
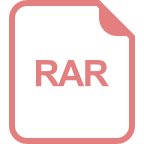
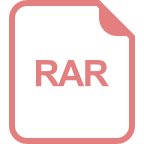
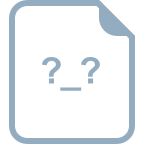














