访问豆瓣电影Top250,在实验内容1的基础上,获取每部电影的导演、编剧、主演、类型、上映时间、片长、评分人数及剧情介绍等信息,并将获取到的信息保存至本地文件中
时间: 2024-06-09 12:11:17 浏览: 252
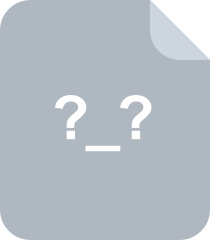
爬取豆瓣电影Top250中的电影信息以及保存相关的数据信息
好的,我将为您提供实现思路。
1. 安装 requests 和 BeautifulSoup 库,用于爬取网页信息和解析网页内容。
2. 分析豆瓣电影Top250的网页结构,找出每个电影对应的链接。
3. 针对每个电影链接,发送请求并解析相应的网页内容,获取需要的电影信息。
4. 将获取到的电影信息保存至本地文件中。
下面是具体实现步骤:
1. 安装 requests 和 BeautifulSoup 库
```
pip install requests
pip install beautifulsoup4
```
2. 分析豆瓣电影Top250的网页结构
豆瓣电影Top250的网页链接为:https://movie.douban.com/top250
打开链接后,可以看到每个电影的信息都在一个链接中,形如:https://movie.douban.com/subject/1292052/
因此,我们需要获取到每个电影链接,然后再针对每个链接进行信息的爬取。
3. 爬取电影信息
针对每个电影链接,发送请求并解析相应的网页内容,获取需要的电影信息。
```
import requests
from bs4 import BeautifulSoup
# 获取页面内容
def get_page(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
response.encoding = 'utf-8'
return response.text
# 解析页面内容,获取电影信息
def parse_page(html):
soup = BeautifulSoup(html, 'html.parser')
# 获取电影标题
title = soup.select_one('h1 > span').text
# 获取电影导演、编剧、主演、类型、上映时间、片长、评分人数及剧情介绍
div_info = soup.select_one('div[id="info"]')
director = div_info.select_one('a[rel="v:directedBy"]').text
screenwriter_list = div_info.select('span[class="attrs"] > a')
screenwriter = '/'.join([s.text for s in screenwriter_list])
actor_list = div_info.select('span[class="actor"] > a')
actor = '/'.join([a.text for a in actor_list])
genre_list = div_info.select('span[property="v:genre"]')
genre = '/'.join([g.text for g in genre_list])
release_date = div_info.select_one('span[property="v:initialReleaseDate"]').text
duration = div_info.select_one('span[property="v:runtime"]').text
rating_count = soup.select_one('span[property="v:votes"]').text
introduction = soup.select_one('span[property="v:summary"]').text.strip()
# 返回电影信息
return {
'title': title,
'director': director,
'screenwriter': screenwriter,
'actor': actor,
'genre': genre,
'release_date': release_date,
'duration': duration,
'rating_count': rating_count,
'introduction': introduction
}
# 爬取电影信息
def spider():
url = 'https://movie.douban.com/top250'
movie_infos = []
# 获取所有电影链接
for i in range(10):
page_url = url + '?start=' + str(i * 25) + '&filter='
page_html = get_page(page_url)
soup = BeautifulSoup(page_html, 'html.parser')
movie_links = soup.select('div[class="hd"] > a')
# 针对每个电影链接,获取电影信息
for link in movie_links:
movie_url = link['href']
movie_html = get_page(movie_url)
movie_info = parse_page(movie_html)
movie_infos.append(movie_info)
# 返回电影信息列表
return movie_infos
# 保存电影信息至本地文件
def save_to_file(movie_infos, filename):
with open(filename, 'w', encoding='utf-8') as f:
for info in movie_infos:
f.write(str(info) + '\n')
# 执行爬虫并保存结果至本地文件
if __name__ == '__main__':
movie_infos = spider()
save_to_file(movie_infos, 'douban_movie_top250.txt')
```
4. 将获取到的电影信息保存至本地文件中
以上代码中,我们定义了 `save_to_file` 函数,用于将获取到的电影信息保存至本地文件中。
在 `spider` 函数中,我们获取到所有的电影信息,并将其存储在 `movie_infos` 列表中。
最后,在 `if __name__ == '__main__'` 中,我们执行爬虫并将结果保存至本地文件 `douban_movie_top250.txt` 中。
完整代码如下:
阅读全文
相关推荐
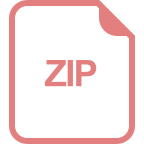


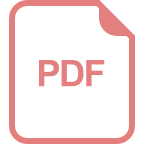
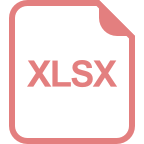
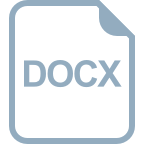
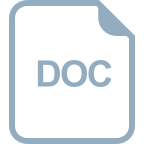




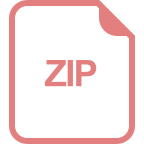
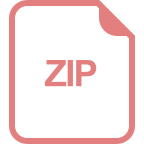
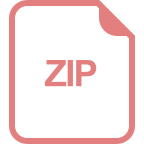
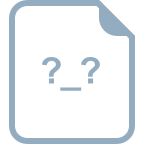
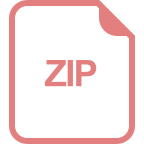