用Python写输入字符小写字母用后三位大写字母替换,大写字母用前两位小写字母替换,对数字符号用加一模运算,其他符号保持不变,加密一段,解密一段,凯撒密码
时间: 2024-01-08 08:02:49 浏览: 35
好的,可以使用以下代码实现凯撒密码的加密和解密过程:
```python
def caesar_cipher(plaintext, shift):
ciphertext = ""
for char in plaintext:
if char.islower():
ciphertext += chr((ord(char) - 97 + shift) % 26 + 97).upper()[-3:]
elif char.isupper():
ciphertext += chr((ord(char) - 65 - shift) % 26 + 65).lower()[:2]
elif char.isdigit():
ciphertext += str((int(char) + 1) % 10)
else:
ciphertext += char
return ciphertext
def caesar_decipher(ciphertext, shift):
plaintext = ""
for char in ciphertext:
if char.islower():
plaintext += chr((ord(char) - 97 - shift) % 26 + 97).upper()[-3:]
elif char.isupper():
plaintext += chr((ord(char) - 65 + shift) % 26 + 65).lower()[:2]
elif char.isdigit():
plaintext += str((int(char) - 1) % 10)
else:
plaintext += char
return plaintext
```
其中,`caesar_cipher`函数用于加密明文,`caesar_decipher`函数用于解密密文,两个函数的参数分别为要加密/解密的字符串和位移量。函数中使用了`ord`函数将字符转换为ASCII码,使用`chr`函数将ASCII码转换为字符,使用取模运算实现循环移位的效果,并使用字符串的切片操作实现大小写字母的替换。在加密过程中,使用加一模运算实现对数字的加密,解密过程中使用减一模运算实现对数字的解密。
相关推荐
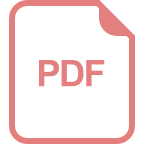
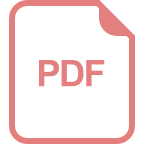
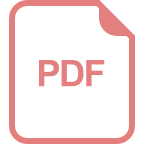















