sklearn one-hot
时间: 2023-10-16 17:07:55 浏览: 79
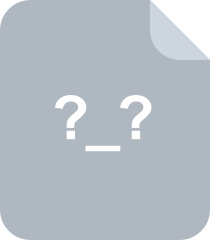
one-hot编码
在scikit-learn中,可以使用OneHotEncoder类来进行独热编码。独热编码是一种将离散特征转换为二进制向量的方法,它将每个可能的值转换为一个数字,然后将其表示为一个向量,其中除了该值索引对应的位置为1,其他位置都为0。这个类可以用于将单个特征或多个特征进行独热编码。可以使用fit_transform()函数来拟合和转换数据,也可以使用transform()函数来对已经拟合好的数据进行转换。需要注意的是,独热编码会增加特征的维度,因此在使用时需要考虑是否需要进行特征选择或者降维处理。
阅读全文
相关推荐
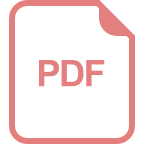


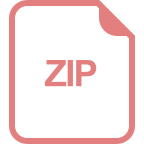
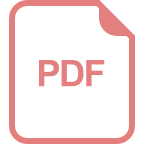












