请帮我写一段使用门控稀疏自编码网络对驾驶行为进行标准化的代码
时间: 2024-04-09 07:28:16 浏览: 7
当然可以!下面是一个使用门控稀疏自编码网络(Gated Sparse Autoencoder,GSAE)对驾驶行为进行标准化的代码示例:
```python
import numpy as np
import tensorflow as tf
# 创建门控稀疏自编码网络类
class GSAE:
def __init__(self, input_dim, hidden_dim, sparsity_ratio):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.sparsity_ratio = sparsity_ratio
self.build_model()
def build_model(self):
self.input = tf.placeholder(tf.float32, shape=[None, self.input_dim])
# 编码层
self.weights_encoder = tf.Variable(tf.random.normal([self.input_dim, self.hidden_dim]))
self.biases_encoder = tf.Variable(tf.random.normal([self.hidden_dim]))
self.encoder = tf.nn.sigmoid(tf.matmul(self.input, self.weights_encoder) + self.biases_encoder)
# 解码层
self.weights_decoder = tf.Variable(tf.random.normal([self.hidden_dim, self.input_dim]))
self.biases_decoder = tf.Variable(tf.random.normal([self.input_dim]))
self.decoder = tf.matmul(self.encoder, self.weights_decoder) + self.biases_decoder
# 稀疏性约束
self.sparsity = tf.reduce_mean(self.encoder, axis=0)
self.kl_divergence = tf.reduce_sum(self.sparsity_ratio * tf.math.log(self.sparsity_ratio / self.sparsity) +
(1 - self.sparsity_ratio) * tf.math.log((1 - self.sparsity_ratio) / (1 - self.sparsity)))
# 损失函数
self.reconstruction_loss = tf.reduce_mean(tf.square(self.input - self.decoder))
self.loss = self.reconstruction_loss + self.kl_divergence
# 优化器
self.optimizer = tf.train.AdamOptimizer().minimize(self.loss)
# 定义训练函数
def train_gsae(data, input_dim, hidden_dim, sparsity_ratio, num_epochs, batch_size):
num_batches = int(data.shape[0] / batch_size)
gsae = GSAE(input_dim, hidden_dim, sparsity_ratio)
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for epoch in range(num_epochs):
np.random.shuffle(data)
for batch in range(num_batches):
batch_data = data[batch * batch_size : (batch + 1) * batch_size]
_, loss = sess.run([gsae.optimizer, gsae.loss], feed_dict={gsae.input: batch_data})
if epoch % 10 == 0:
print("Epoch:", epoch, "Loss:", loss)
# 返回训练好的编码器权重和偏置
weights_encoder, biases_encoder = sess.run([gsae.weights_encoder, gsae.biases_encoder])
return weights_encoder, biases_encoder
# 使用示例
input_dim = 100
hidden_dim = 50
sparsity_ratio = 0.1
num_epochs = 100
batch_size = 32
# 假设有驾驶行为数据集data,形状为(num_samples, input_dim)
data = np.random.rand(1000, input_dim)
weights_encoder, biases_encoder = train_gsae(data, input_dim, hidden_dim, sparsity_ratio, num_epochs, batch_size)
# 使用训练好的编码器对新数据进行标准化
new_data = np.random.rand(10, input_dim)
normalized_data = np.sigmoid(np.matmul(new_data, weights_encoder) + biases_encoder)
```
这段代码使用TensorFlow实现了一个简单的门控稀疏自编码网络。训练函数`train_gsae`接受驾驶行为数据集`data`,输入维度`input_dim`,隐藏层维度`hidden_dim`,稀疏比例`sparsity_ratio`,训练周期数`num_epochs`和批量大小`batch_size`作为输入,并返回训练好的编码器权重和偏置。然后,可以使用训练好的编码器对新数据进行标准化。
相关推荐
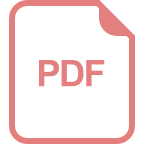
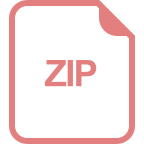














