python正则匹配url中的参数
时间: 2024-05-09 09:20:42 浏览: 20
使用正则表达式来匹配URL中的参数:
```
import re
url = 'https://www.example.com/search?q=python&sort=desc'
# 匹配参数
params = re.findall(r'\?(\w+)=(\w+)', url)
# 输出参数
for param in params:
print(param[0], param[1])
```
上述代码将输出:
```
q python
sort desc
```
解释:
- `\?`:匹配问号
- `(\w+)`:匹配参数名,使用括号捕获分组
- `=`:匹配等号
- `(\w+)`:匹配参数值,使用括号捕获分组
此外,如果需要匹配多个URL,可以使用正则表达式的`re.findall()`方法来匹配多个URL。
相关问题
python 正则匹配url中的域名
可以使用Python中的re模块来进行正则匹配。下面是一个例子:
```python
import re
url = "https://www.baidu.com/s?wd=python"
# 使用正则表达式匹配域名
pattern = r"(https?://)?(www\.)?([a-zA-Z0-9-]+)\.[a-zA-Z]+(/[a-zA-Z0-9-]*)*"
match = re.search(pattern, url)
if match:
domain = match.group(3)
print(domain)
else:
print("No domain found in the URL.")
```
在上面的代码中,我们使用正则表达式`"(https?://)?(www\.)?([a-zA-Z0-9-]+)\.[a-zA-Z]+(/[a-zA-Z0-9-]*)*"`来匹配URL中的域名。其中,`([a-zA-Z0-9-]+)\.[a-zA-Z]+`匹配域名部分,`([a-zA-Z0-9-]+)`表示域名中可以包含字母、数字和横线,`\.`表示匹配点号,`[a-zA-Z]+`表示匹配顶级域名,例如`.com`、`.cn`等。`(/[a-zA-Z0-9-]*)*`表示匹配URL中的路径部分,用于处理类似于`https://www.baidu.com/s`这样的URL。如果匹配成功,则输出域名部分。
python 正则匹配url
你可以使用正则表达式来匹配URL。下面是一个示例的Python代码:
```python
import re
def match_url(string):
pattern = r'https?://(?:[-\w.]|(?:%[\da-fA-F]{2}))+'
matches = re.findall(pattern, string)
return matches
# 示例用法
text = "This is an example string with URLs like https://www.example.com and http://example.com"
urls = match_url(text)
print(urls)
```
这段代码将输出匹配到的URL,如 `['https://www.example.com', 'http://example.com']`。则表达式 `r'https?://(?:[-\w.]|(?:%[\da-fA-F]{2}))+` 可以用来匹配符合URL格式的字符串。
相关推荐
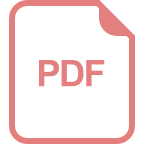
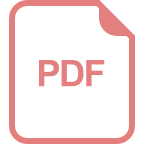












