搭建一个pytest 接口测试框架
时间: 2023-02-07 11:37:17 浏览: 181
好的,下面是搭建 PyTest 接口测试框架的步骤:
1. 安装 PyTest。在命令行中输入 `pip install pytest` 即可安装。
2. 创建一个测试用例文件。通常来说,测试用例文件名都以 `test_` 开头,如 `test_login.py`。
3. 编写测试用例。测试用例通常是一个函数,以 `test_` 开头,例如 `def test_login():`。
4. 在测试用例函数中编写测试代码。测试代码中应该包含请求接口、获取响应数据和断言的过程。
5. 运行测试用例。在命令行中输入 `pytest`,就可以运行所有以 `test_` 开头的测试用例了。
希望这些步骤能够帮到你。如果你有其他问题,欢迎继续提问。
相关问题
搭建一个pytest+allure+request接口自动化框架
好的,这里为您提供一个简单的pytest+allure+requests接口自动化框架搭建步骤:
1. 安装pytest和allure
```
pip install pytest
pip install allure-pytest
```
2. 安装requests库
```
pip install requests
```
3. 创建测试用例文件和测试数据文件
在项目中创建一个tests文件夹,用于存放测试用例文件。在tests文件夹中创建一个test_api.py文件,用于编写测试用例。
同时,也需要在项目中创建一个data文件夹,用于存放测试数据文件。在data文件夹中创建一个api_data.json文件,用于存放接口测试数据。
4. 编写测试用例
在test_api.py文件中,使用pytest编写测试用例。可以使用requests库发送接口请求,并对返回结果进行断言。
示例代码:
```python
import pytest
import requests
class TestAPI:
@pytest.fixture(scope='session', autouse=True)
def setup_class(self):
self.base_url = 'https://api.github.com'
def test_get_user(self):
url = self.base_url + '/users/github'
response = requests.get(url)
assert response.status_code == 200
assert response.json()['login'] == 'github'
def test_create_gist(self):
url = self.base_url + '/gists'
data = {
"description": "test gist",
"public": True,
"files": {
"test.txt": {
"content": "Test gist content"
}
}
}
headers = {
"Authorization": "token <your access token>"
}
response = requests.post(url, json=data, headers=headers)
assert response.status_code == 201
```
5. 编写测试数据
在api_data.json文件中,编写接口测试用到的数据。可以对不同接口的测试数据进行分类,方便维护。
示例代码:
```json
{
"get_user": {
"url": "/users/github",
"method": "get",
"headers": {},
"params": {},
"data": {},
"json": {},
"expected_status_code": 200,
"expected_data": {
"login": "github"
}
},
"create_gist": {
"url": "/gists",
"method": "post",
"headers": {
"Authorization": "token <your access token>"
},
"params": {},
"data": {
"description": "test gist",
"public": true,
"files": {
"test.txt": {
"content": "Test gist content"
}
}
},
"json": {},
"expected_status_code": 201,
"expected_data": {}
}
}
```
6. 使用pytest执行测试用例
在项目根目录下打开终端,执行如下命令:
```
pytest --alluredir=./report
```
该命令将会执行tests文件夹下的所有测试用例,并将测试结果保存在report文件夹中。
7. 生成测试报告
执行完测试用例后,使用如下命令生成测试报告:
```
allure generate ./report -o ./report/html --clean
```
该命令将会在report文件夹下生成一个html文件夹,里面包含测试结果的详细信息和图表。
至此,一个简单的pytest+allure+requests接口自动化框架就搭建完成了。
帮我搭建一个简单的pytest接口自动化框架+allure
要搭建一个简单的pytest接口自动化测试框架并集成Allure,你需要做以下几个步骤:
1. **安装Python环境**:首先确保你的环境中已经安装了Python和pip,用于管理包。
2. **选择测试框架**:`pytest`是一个流行的Python测试框架,它专注于简洁、快速和易于使用。
3. **安装pytest和相关库**:
- 安装pytest: `pip install pytest`
- 安装requests库,用于发送HTTP请求: `pip install requests`
- 可选安装fixture(预设条件)支持:如`pytest-flask`如果测试的是Flask应用,或者`pytest-httpx`如果需要处理HTTPS请求。
4. **创建测试模块**:创建一个名为`test_api.py`的文件,开始编写测试用例。例如,你可以创建一个方法来模拟API请求:
```python
import pytest
import requests
def test_get_status_code():
response = requests.get('http://your-api-url')
assert response.status_code == 200
```
5. **配置Allure报告**:
- 安装Allure-Python-Report-Generator:`pip install allure-python-report-generator`
- 在`pytest.ini`或`setup.cfg`文件中添加Allure插件配置:
```ini
[pytest]
plugins = allure
addopts = --alluredir=allure-results
```
6. **启用Allure报告**:在每个测试函数前添加装饰器`@pytest.mark.allure`,以标记哪些测试将被Allure记录:
```python
@pytest.mark.allure
def test_post_data():
response = requests.post('http://your-api-url', data={'key': 'value'})
allure.attach(response.text, name='response_body', attachment_type=allure.attachment_type.TEXT)
assert response.status_code == 201
```
7. **运行测试**:运行pytest命令,它会生成Allure报告。`pytest -v`可查看详细信息。
阅读全文
相关推荐
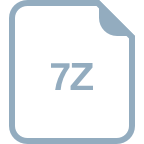
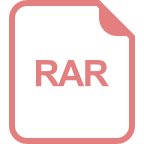
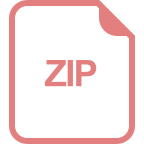
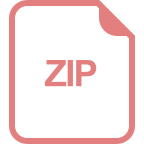
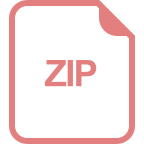
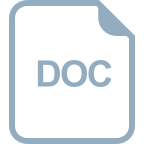
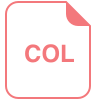





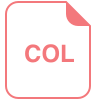
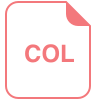
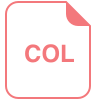