设计一个目标检测的UI界面的代码
时间: 2023-12-03 16:46:59 浏览: 55
以下是一个简单的目标检测UI界面代码示例,使用Python和Tkinter GUI库:
```python
import tkinter as tk
from PIL import ImageTk, Image
import cv2
# 加载模型和标签
model = cv2.dnn.readNetFromTensorflow('frozen_inference_graph.pb', 'graph.pbtxt')
labels = open('labels.txt').read().strip().split('\n')
# 创建主窗口
root = tk.Tk()
root.title("目标检测")
# 创建画布
canvas = tk.Canvas(root, width=700, height=500)
canvas.pack()
# 创建标签和按钮
label = tk.Label(root, text="选择图片进行目标检测")
label.pack(side=tk.TOP, pady=10)
browse_button = tk.Button(root, text="浏览", command=lambda: browse_image())
browse_button.pack(side=tk.TOP, pady=10)
detect_button = tk.Button(root, text="检测", command=lambda: detect_objects())
detect_button.pack(side=tk.TOP, pady=10)
# 浏览图片函数
def browse_image():
global img_path
img_path = tk.filedialog.askopenfilename()
img = Image.open(img_path)
img = img.resize((500, 400), Image.ANTIALIAS)
img = ImageTk.PhotoImage(img)
canvas.img = img
canvas.create_image(0, 0, anchor='nw', image=img)
# 目标检测函数
def detect_objects():
# 加载图片
image = cv2.imread(img_path)
# 获取图片大小
(h, w) = image.shape[:2]
# 构建一个 blob 对象
blob = cv2.dnn.blobFromImage(cv2.resize(image, (300, 300)), 0.007843, (300, 300), 127.5)
# 设置输入
model.setInput(blob)
# 运行前向传递以检测目标
detections = model.forward()
# 循环检测结果
for i in range(0, detections.shape[2]):
# 提取置信度
confidence = detections[0, 0, i, 2]
# 过滤掉低置信度检测结果
if confidence > 0.5:
# 计算边界框坐标
box = detections[0, 0, i, 3:7] * np.array([w, h, w, h])
(startX, startY, endX, endY) = box.astype("int")
# 提取类别 ID
classID = int(detections[0, 0, i, 1])
# 绘制边界框和标签
label = "{}: {:.2f}%".format(labels[classID], confidence * 100)
cv2.rectangle(image, (startX, startY), (endX, endY), (0, 255, 0), 2)
y = startY - 15 if startY - 15 > 15 else startY + 15
cv2.putText(image, label, (startX, y), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 将结果显示在 Tkinter 画布上
image = Image.fromarray(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
image = image.resize((500, 400), Image.ANTIALIAS)
img = ImageTk.PhotoImage(image)
canvas.img = img
canvas.create_image(0, 0, anchor='nw', image=img)
# 运行主循环
root.mainloop()
```
注意,此代码仅是一个示例,并且需要根据您的具体需求进行修改和优化。
相关推荐
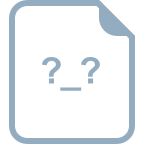
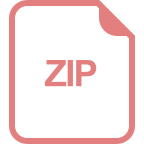














