flutter 验证码图片算法
时间: 2023-11-25 08:05:47 浏览: 30
Flutter 并没有提供专门的验证码图片算法,但可以使用 Flutter 提供的图形绘制 API 和随机数生成 API 来实现验证码图片算法。
以下是一个简单的示例代码,实现了一个四位数的数字验证码图片生成:
```dart
import 'dart:math';
import 'dart:ui';
import 'package:flutter/material.dart';
class CaptchaImage extends StatefulWidget {
final ValueChanged<String> onCaptchaChanged;
CaptchaImage({Key key, this.onCaptchaChanged}) : super(key: key);
@override
_CaptchaImageState createState() => _CaptchaImageState();
}
class _CaptchaImageState extends State<CaptchaImage> {
String captcha;
final TextEditingController _textEditingController =
TextEditingController();
@override
void initState() {
super.initState();
_generateCaptcha();
}
void _generateCaptcha() {
setState(() {
captcha = _generateRandomString(4); // 生成四个随机数字
widget.onCaptchaChanged?.call(captcha);
});
}
String _generateRandomString(int length) {
var random = Random.secure();
var values = List<int>.generate(length, (i) => random.nextInt(10));
return values.join();
}
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: _generateCaptcha,
child: CustomPaint(
size: Size(100, 40),
painter: CaptchaPainter(captcha),
),
);
}
}
class CaptchaPainter extends CustomPainter {
final String captcha;
final Paint _paint = Paint()
..color = Colors.black
..strokeWidth = 1.5
..style = PaintingStyle.stroke;
CaptchaPainter(this.captcha);
@override
void paint(Canvas canvas, Size size) {
// 绘制背景
canvas.drawRect(Rect.fromLTWH(0, 0, size.width, size.height),
Paint()..color = Colors.white);
// 绘制验证码
var textStyle = TextStyle(fontSize: 24, fontWeight: FontWeight.bold);
var textSpan = TextSpan(
text: captcha,
style: textStyle,
);
var textPainter = TextPainter(
text: textSpan,
textAlign: TextAlign.center,
textDirection: TextDirection.ltr,
);
textPainter.layout(minWidth: 0, maxWidth: size.width);
textPainter.paint(canvas, Offset((size.width - textPainter.width) / 2, 8));
// 绘制干扰线
var random = Random.secure();
for (int i = 0; i < 4; i++) {
var startX = random.nextInt(size.width.toInt());
var startY = random.nextInt(size.height.toInt());
var endX = random.nextInt(size.width.toInt());
var endY = random.nextInt(size.height.toInt());
canvas.drawLine(Offset(startX.toDouble(), startY.toDouble()),
Offset(endX.toDouble(), endY.toDouble()), _paint);
}
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return true;
}
}
```
这个示例代码中,`CaptchaImage` 是一个自定义的 `StatefulWidget`,用来生成验证码图片。`CaptchaPainter` 是一个自定义的 `CustomPainter`,用来绘制验证码图片。在 `CaptchaPainter` 中,我们先绘制一个白色的矩形作为背景,然后使用 `TextPainter` 绘制随机生成的四个数字,最后再绘制四条随机干扰线。在 `CaptchaImage` 中,我们通过 `CustomPaint` 将 `CaptchaPainter` 绘制出来,并且通过 `GestureDetector` 来响应用户点击事件,生成新的验证码。
相关推荐
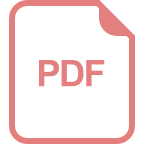
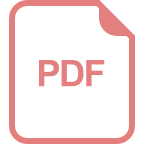
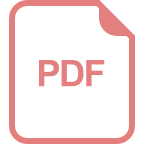














