用C++代码写出两条直线的角度公式
时间: 2024-05-24 14:13:43 浏览: 135
我可以回答这个问题。两条直线的角度公式可以通过向量的点积来计算。假设直线1的向量为(a1, b1),直线2的向量为(a2, b2),则它们的夹角可以使用以下公式计算:
angle = arccos((a1 * a2 + b1 * b2) / (sqrt(a1^2 + b1^2) * sqrt(a2^2 + b2^2)))
其中,arccos表示反余弦函数,可以使用数学库中的函数来计算。
相关问题
用C++代码 写出 两直线夹角角度
我可以回答这个问题。要计算两条直线的夹角,可以使用向量的内积公式,即两条直线的向量点积除以它们的长度乘积,再用反余弦函数求出夹角的弧度值,最后将弧度值转化为角度。以下是示例代码:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
double x;
double y;
} Vector;
double dot(Vector v1, Vector v2)
{
return v1.x * v2.x + v1.y * v2.y;
}
double length(Vector v)
{
return sqrt(v.x * v.x + v.y * v.y);
}
double angle(Vector v1, Vector v2)
{
double cos_theta = dot(v1, v2) / (length(v1) * length(v2));
double theta = acos(cos_theta);
return theta * 180 / M_PI;
}
int main()
{
Vector v1 = {1, 2};
Vector v2 = {-3, 4};
double theta = angle(v1, v2);
printf("The angle between (%g, %g) and (%g, %g) is %g degrees.\n",
v1.x, v1.y, v2.x, v2.y, theta);
return 0;
}
```
输出结果为:
```
The angle between (1, 2) and (-3, 4) is 126.869 degrees.
```
c++ 已知两条直线的首尾两点,请写出两条直线的平分线完整计算过程
在 C++ 中,如果你已经知道了两条直线的首尾两点 (PointA1, PointB1) 和 (PointA2, PointB2),计算这两条直线的交点,即它们的平分线上的一个点,通常需要找到它们斜率相等的情况。因为如果两条线平行,则没有交点;如果它们垂直,则交点就是两线段的中点。
首先,你需要确定两条直线是否垂直。为此,计算每条直线的斜率:
对于第一条直线:
```cpp
double slope1 = (PointB1.y - PointA1.y) / (PointB1.x - PointA1.x);
```
对于第二条直线:
```cpp
double slope2 = (PointB2.y - PointA2.y) / (PointB2.x - PointA2.x);
```
然后判断斜率是否相等:
```cpp
bool areParallel = abs(slope1 - slope2) < std::numeric_limits<double>::epsilon(); // 判断接近于0
```
如果 `areParallel` 为 true,说明两条直线平行,直接返回其中一条线的中点作为“平分线”:
```cpp
if (areParallel) {
double midX = (PointA1.x + PointB1.x) / 2;
double midY = (PointA1.y + PointB1.y) / 2;
return {midX, midY}; // 返回中点坐标
}
```
如果斜率不相等,那么两条线有交点。可以将求解两个方程组的问题转化为一次消元法或者解析几何的方法。假设斜率不等于0,我们可以先转换成点斜式公式找到两条直线的交点 P(x, y):
设交点P(x, y),则有:
- 对于直线1:y = slope1 * (x - PointA1.x) + PointA1.y
- 对于直线2:y = slope2 * (x - PointA2.x) + PointA2.y
令这两个方程相等,解出 x:
```cpp
double xIntersection = (slope2 * PointA1.x - slope1 * PointA2.x + PointA2.y - PointA1.y) / (slope1 - slope2);
double yIntersection = slope1 * (xIntersection - PointA1.x) + PointA1.y; // 计算对应的y值
```
最后得到交点坐标 (xIntersection, yIntersection) 即为所求的平分线上的点。
阅读全文
相关推荐
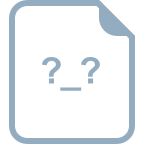

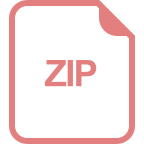
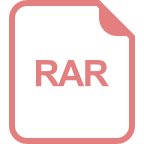
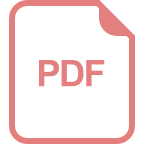
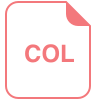
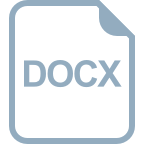
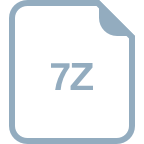
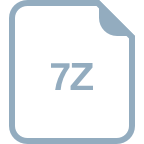
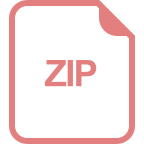
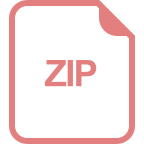