2. 编写一个程序,程序实现对用户指定的文本文件中的英文字符和字符串的个数进行统计的功能,并将结果根据用户选择输出至结果文件或屏幕。 1) 构建统计类,该类实现对I/O的操纵;实现对文本文件中英文字符、字符串的统计;实现对统计结果的输出。 2) 构建测试类,该类实现与用户的交互,向用户提示操作信息,并接收用户的操作请求。 程序应具有良好的人机交互性能,即:程序应向用户提示功能说明,并可根据用户的功能选择,执行对应的功能,并给出带详细描述信息的最终执行结果。
时间: 2024-02-25 08:58:44 浏览: 7
好的,这是一个比较典型的Java上机作业,下面我给您提供一个基本的实现思路:
1. 创建一个统计类,该类实现对I/O的操纵,实现对文本文件中英文字符、字符串的统计,实现对统计结果的输出。
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class TextFileStatistics {
private File file;
private String outputPath;
private int totalCharCount;
private int totalStringCount;
public TextFileStatistics(File file, String outputPath) {
this.file = file;
this.outputPath = outputPath;
}
public void process() throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
totalCharCount += line.length();
String[] words = line.split(" ");
totalStringCount += words.length;
}
reader.close();
}
public void output() throws IOException {
FileWriter writer = new FileWriter(outputPath);
writer.write("Total character count: " + totalCharCount + "\n");
writer.write("Total string count: " + totalStringCount + "\n");
writer.close();
}
}
```
2. 创建一个测试类,该类实现与用户的交互,向用户提示操作信息,并接收用户的操作请求。
```java
import java.io.File;
import java.io.IOException;
import java.util.Scanner;
public class TextFileStatisticsTest {
public static void main(String[] args) throws IOException {
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter the input file path: ");
String inputPath = scanner.nextLine();
File inputFile = new File(inputPath);
while (!inputFile.exists()) {
System.out.print("Invalid path, please enter again: ");
inputPath = scanner.nextLine();
inputFile = new File(inputPath);
}
System.out.print("Please enter the output file path (or type 'console' to output to console): ");
String outputPath = scanner.nextLine();
while (!outputPath.equals("console") && new File(outputPath).exists()) {
System.out.print("File already exists, do you want to overwrite it? (y/n): ");
String choice = scanner.nextLine();
if (choice.equals("y")) {
break;
} else {
System.out.print("Please enter another path: ");
outputPath = scanner.nextLine();
}
}
TextFileStatistics statistics = new TextFileStatistics(inputFile, outputPath);
statistics.process();
if (outputPath.equals("console")) {
statistics.outputToConsole();
} else {
statistics.output();
}
scanner.close();
}
}
```
3. 在测试类中,通过Scanner类获取用户输入的文件路径和输出路径,然后创建TextFileStatistics对象,调用process()方法进行统计,最后根据用户选择输出到控制台或文件中。
这是一个基本的实现思路,您可以根据自己的需要修改和完善代码。
相关推荐
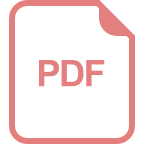
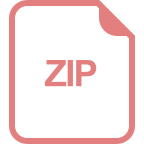
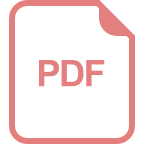













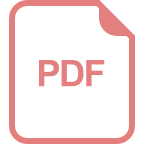