java代码实现. 编写一个程序,程序实现对用户指定的文本文件中的英文字符和字符串 的个数进行统计的功能,并将结果根据用户选择输出至结果文件或屏幕。 第 5 / 6 页 1) 构建统计类,该类实现对 I/O 的操纵;实现对文本文件中英文字符、字符 串的统计;实现对统计结果的输出。 2) 构建测试类,该类实现与用户的交互,向用户提示操作信息,并接收用 户的操作请求。
时间: 2024-02-18 10:01:21 浏览: 24
下面是一个简单的 Java 代码实现,用于统计指定文本文件中的英文字符和字符串的个数,并将结果输出到屏幕或结果文件中:
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class TextFileAnalyzer {
// 统计类
private static class Analyzer {
private int charCount;
private int wordCount;
// 统计英文字符和字符串个数
public void analyze(File file) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
// 统计字符数
charCount += line.length();
// 统计单词数
String[] words = line.split("\\s+");
wordCount += words.length;
}
reader.close();
}
// 输出统计结果
public void output(boolean toFile, String fileName) throws IOException {
if (toFile) {
FileWriter writer = new FileWriter(fileName);
writer.write("字符数:" + charCount + "\n");
writer.write("单词数:" + wordCount + "\n");
writer.close();
} else {
System.out.println("字符数:" + charCount);
System.out.println("单词数:" + wordCount);
}
}
}
// 测试类
public static void main(String[] args) throws IOException {
Analyzer analyzer = new Analyzer();
// 从命令行参数中读取文件名
if (args.length == 0) {
System.out.println("请指定要统计的文件名");
return;
}
File file = new File(args[0]);
if (!file.exists()) {
System.out.println("指定的文件不存在");
return;
}
// 与用户交互,选择输出方式
System.out.println("请选择输出方式:");
System.out.println("1. 输出到屏幕");
System.out.println("2. 输出到文件");
int choice = System.in.read() - '0';
switch (choice) {
case 1:
analyzer.analyze(file);
analyzer.output(false, null);
break;
case 2:
System.out.println("请输入结果文件名:");
String fileName = new BufferedReader(new java.io.InputStreamReader(System.in)).readLine();
analyzer.analyze(file);
analyzer.output(true, fileName);
break;
default:
System.out.println("无效的选项");
break;
}
}
}
```
在本程序中,我们使用了一个内部类 `Analyzer` 来实现文本文件的分析和统计。在 `analyze` 方法中,我们使用 `BufferedReader` 来逐行读取文本文件,然后通过 `line.length()` 统计字符数,通过 `line.split("\\s+")` 来将每行文本按空格拆分成单词,并统计单词数。最后,在 `output` 方法中,我们根据用户选择将结果输出到屏幕或结果文件中。
在测试类中,我们首先从命令行参数中读取要统计的文件名,然后与用户交互,让用户选择输出方式。如果用户选择将结果输出到文件中,我们还要让用户输入结果文件名。最后,我们调用 `Analyzer` 类的方法进行文本文件的分析和统计,并根据用户选择将结果输出到屏幕或结果文件中。
相关推荐
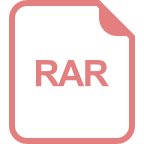
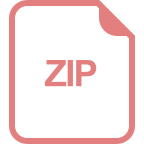












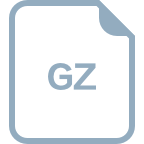
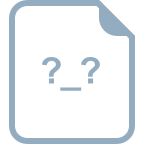