请用Java写一个程序满足以下要求:2. 编写一个程序,程序实现对用户指定的文本文件中的英文字符和字符串的个数进行统计的功能,并将结果根据用户选择输出至结果文件或屏幕。 1)构建统计类,该类实现对I/O的操纵;实现对文本文件中英文字符、字符串的统计;实现对统计结果的输出。 构建测试类,该类实现与用户的交互,向用户提示操作信息,并接收用户的操作请求。
时间: 2023-07-15 08:14:08 浏览: 75
以下是一个简单的实现:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
public class TextFileStatistics {
private String filePath;
private int charCount;
private int wordCount;
private int lineCount;
public TextFileStatistics(String filePath) {
this.filePath = filePath;
charCount = 0;
wordCount = 0;
lineCount = 0;
}
public void count() throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(filePath));
String line;
while ((line = reader.readLine()) != null) {
lineCount++;
charCount += line.length();
wordCount += line.split("\\s+").length;
}
reader.close();
}
public void output(boolean toScreen, String resultFilePath) throws IOException {
if (toScreen) {
System.out.println("Character count: " + charCount);
System.out.println("Word count: " + wordCount);
System.out.println("Line count: " + lineCount);
} else {
PrintWriter writer = new PrintWriter(new FileWriter(resultFilePath));
writer.println("Character count: " + charCount);
writer.println("Word count: " + wordCount);
writer.println("Line count: " + lineCount);
writer.close();
}
}
}
```
测试类:
```java
import java.io.IOException;
import java.util.Scanner;
public class TestTextFileStatistics {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the file path: ");
String filePath = scanner.nextLine();
TextFileStatistics statistics = new TextFileStatistics(filePath);
try {
statistics.count();
System.out.print("Output to screen? (y/n): ");
boolean toScreen = scanner.nextLine().equalsIgnoreCase("y");
if (toScreen) {
statistics.output(true, null);
} else {
System.out.print("Enter the result file path: ");
String resultFilePath = scanner.nextLine();
statistics.output(false, resultFilePath);
}
System.out.println("Counting completed.");
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
} finally {
scanner.close();
}
}
}
```
注意事项:
- 以上代码只能正确统计 ASCII 码中的英文字符,如果需要统计其它字符,需要根据具体需求进行修改;
- 以上代码只能正确统计单词数量,如果需要统计其它语法单位数量,需要根据具体需求进行修改;
- 以上代码没有对文件路径合法性进行判断,需要根据具体需求进行修改。
阅读全文
相关推荐
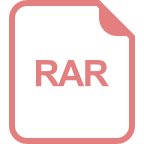
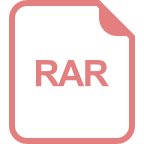
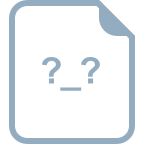
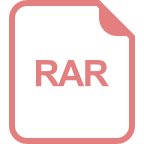
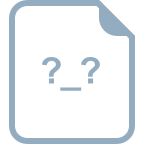
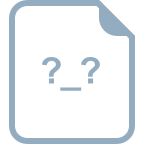
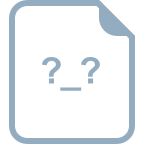
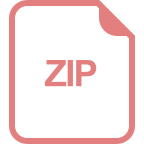
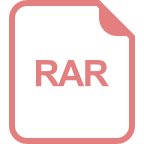
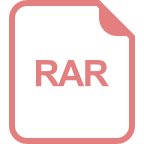
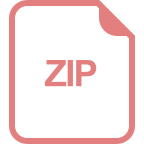
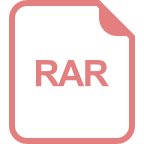
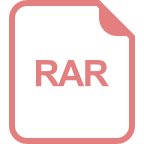
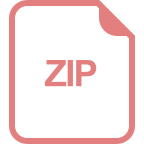
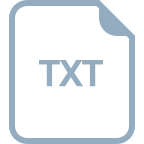
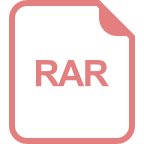
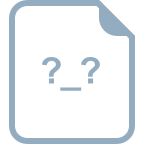