java编写一个程序,实现对用户指定的文本文件中的英文字符和字符串的个数进行统计的功能,实现对I/O的操纵
时间: 2024-02-11 10:09:09 浏览: 76
好的,以下是一个Java程序,可以实现对用户指定的文本文件中的英文字符和字符串的个数进行统计的功能,并且涉及了I/O的操纵。
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class FileAnalyzer {
public static void main(String[] args) throws IOException {
// 获取用户指定的文本文件路径
String filePath = args[0];
// 创建一个File对象
File file = new File(filePath);
// 创建一个BufferedReader对象,用于读取文件内容
BufferedReader reader = new BufferedReader(new FileReader(file));
// 初始化字符和字符串计数器
int charCount = 0;
int wordCount = 0;
// 定义一个字符串变量,用于存储每行读取的内容
String line;
// 逐行读取文件内容,直到读到最后一行
while ((line = reader.readLine()) != null) {
// 更新字符计数器,统计每行中的英文字符个数
charCount += countChars(line);
// 更新字符串计数器,统计每行中的英文字符串个数
wordCount += countWords(line);
}
// 关闭文件读取器
reader.close();
// 输出统计结果
System.out.println("英文字符数:" + charCount);
System.out.println("英文字符串数:" + wordCount);
}
/**
* 统计指定字符串中的英文字符个数
* @param str 指定字符串
* @return 英文字符个数
*/
private static int countChars(String str) {
int count = 0;
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
if ((c >= 'A' && c <= 'Z') || (c >= 'a' && c <= 'z')) {
// 如果是英文字母,则计数器加1
count++;
}
}
return count;
}
/**
* 统计指定字符串中的英文单词个数
* @param str 指定字符串
* @return 英文单词个数
*/
private static int countWords(String str) {
int count = 0;
String[] words = str.trim().split("\\s+");
for (String word : words) {
if (word.matches("[A-Za-z]+")) {
// 如果是英文单词,则计数器加1
count++;
}
}
return count;
}
}
```
这个程序的基本思路是:读取用户指定的文本文件,逐行统计每行中的英文字符和英文单词数,并将统计结果输出到控制台。具体实现细节如下:
1. 通过命令行参数获取用户指定的文本文件路径。
2. 创建一个File对象,用于表示指定路径的文本文件。
3. 创建一个BufferedReader对象,用于读取文本文件内容。
4. 初始化字符和字符串计数器。
5. 逐行读取文本文件内容,并在每行中统计英文字符和英文单词个数。
6. 关闭文件读取器。
7. 输出统计结果。
阅读全文
相关推荐














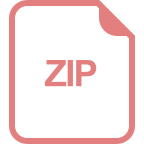