1. 编写一个程序,程序实现对用户指定的文本文件中的英文字符和字符串 的个数进行统计的功能,并将结果根据用户选择输出至结果文件或屏幕。 第 5 / 6 页 1) 构建统计类,该类实现对 I/O 的操纵;实现对文本文件中英文字符、字符 串的统计;实现对统计结果的输出。 2) 构建测试类,该类实现与用户的交互,向用户提示操作信息,并接收用 户的操作请求。 程序应具有良好的人机交互性能,即:程序应向用户提示功能说明,并可根 据用户的功能选择,执行对应的功能,并给出带详细描述信息的最终执行结果。
时间: 2024-02-17 21:04:40 浏览: 19
好的,可以的。这是一个比较典型的程序设计任务,需要实现类的设计和交互式用户界面的设计。我可以帮你解答相关问题和提供思路,但是具体代码实现需要你自己完成。
首先,你需要设计一个统计类,这个类应该包含以下成员变量和方法:
- 成员变量:输入文件路径、统计结果、输出方式(结果文件路径或屏幕输出)等。
- 方法:从文件中读取英文字符和字符串,统计英文字符和字符串的个数,将结果输出至结果文件或屏幕。
以下是一个可能的统计类的代码示例(请注意这只是一个示例,具体实现可能还需要进行修改和调整):
```python
import os
class TextStatistics:
def __init__(self):
self.input_file_path = ''
self.result = {}
self.output_mode = 0
self.output_file_path = ''
def set_input_file_path(self, file_path):
self.input_file_path = file_path
def set_output_mode(self, mode):
self.output_mode = mode
def set_output_file_path(self, file_path):
self.output_file_path = file_path
def count(self):
if not os.path.exists(self.input_file_path):
print('Input file does not exist')
return
with open(self.input_file_path, 'r') as f:
text = f.read()
# 统计英文字符个数
char_count = 0
for char in text:
if char.isalpha():
char_count += 1
# 统计英文字符串个数
word_count = 0
words = text.split()
for word in words:
if word.isalpha():
word_count += 1
self.result = {
'char_count': char_count,
'word_count': word_count
}
def output(self):
if self.output_mode == 0:
print(self.result)
else:
with open(self.output_file_path, 'w') as f:
f.write(str(self.result))
```
接下来,你需要设计一个测试类,这个类应该包含以下成员变量和方法:
- 成员变量:统计类对象、用户输入的选项。
- 方法:向用户提示操作信息,接收用户的操作请求,并调用统计类对象的方法进行操作。
以下是一个可能的测试类的代码示例(请注意这只是一个示例,具体实现可能还需要进行修改和调整):
```python
class TextStatisticsTest:
def __init__(self):
self.statistics = TextStatistics()
def run(self):
while True:
print('Please select an option:')
print('1. Set input file path')
print('2. Set output mode')
print('3. Set output file path')
print('4. Count')
print('5. Quit')
option = input('Enter your option: ')
if option == '1':
file_path = input('Enter input file path: ')
self.statistics.set_input_file_path(file_path)
elif option == '2':
mode = input('Enter output mode (0 for screen, 1 for file): ')
self.statistics.set_output_mode(int(mode))
elif option == '3':
file_path = input('Enter output file path: ')
self.statistics.set_output_file_path(file_path)
elif option == '4':
self.statistics.count()
self.statistics.output()
elif option == '5':
break
else:
print('Invalid option')
```
你可以根据具体情况进行修改和调整,比如增加对用户输入的校验等。最后,在主程序中创建测试类对象并运行即可:
```python
if __name__ == '__main__':
test = TextStatisticsTest()
test.run()
```
相关推荐
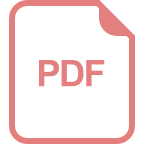
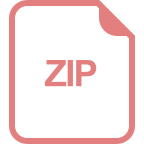
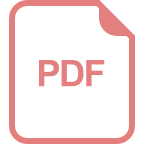












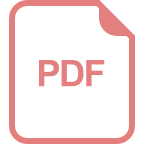