使用C语言实现上述功能
时间: 2023-07-20 14:26:39 浏览: 40
好的,这里提供一个使用C语言实现上述功能的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define CONFIG_FILE "config.ini"
int main() {
// 定义默认的配置参数
int reboot_count = 0;
int reboot_status = 0;
int reboot_flag = -1;
int image_cnt = 0;
// 检查配置文件是否存在,如果不存在则创建
if (access(CONFIG_FILE, F_OK) == -1) {
// 创建配置文件
FILE *fp = fopen(CONFIG_FILE, "w");
if (fp == NULL) {
printf("Failed to create config file!\n");
return -1;
}
// 写入默认的配置参数
fprintf(fp, "[DEFAULT]\n");
fprintf(fp, "reboot_count=%d\n", reboot_count);
fprintf(fp, "reboot_status=%d\n", reboot_status);
fprintf(fp, "reboot_flag=%d\n", reboot_flag);
fprintf(fp, "image_cnt=%d\n", image_cnt);
// 关闭文件
fclose(fp);
} else {
// 如果配置文件存在,则直接打开
FILE *fp = fopen(CONFIG_FILE, "r");
if (fp == NULL) {
printf("Failed to open config file!\n");
return -1;
}
// 读取配置参数
char line[100];
while (fgets(line, sizeof(line), fp)) {
int value;
if (sscanf(line, "reboot_count=%d", &value) == 1) {
reboot_count = value;
} else if (sscanf(line, "reboot_status=%d", &value) == 1) {
reboot_status = value;
} else if (sscanf(line, "reboot_flag=%d", &value) == 1) {
reboot_flag = value;
} else if (sscanf(line, "image_cnt=%d", &value) == 1) {
image_cnt = value;
}
}
// 关闭文件
fclose(fp);
}
// 打印读取到的配置参数
printf("reboot_count=%d\n", reboot_count);
printf("reboot_status=%d\n", reboot_status);
printf("reboot_flag=%d\n", reboot_flag);
printf("image_cnt=%d\n", image_cnt);
return 0;
}
```
上面的代码中,我们同样是先定义了默认的配置参数,然后通过检查配置文件是否存在来决定是创建还是打开配置文件。如果配置文件不存在,则创建一个新的配置文件,并将默认的配置参数写入其中。如果配置文件已经存在,则直接打开该配置文件,并读取其中的参数值。最后,我们打印读取到的配置参数,以便检查是否正确读取。
希望这个C语言的示例代码可以帮助到你。如果你还有其他的问题,请继续提问。
相关推荐
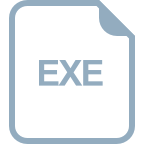
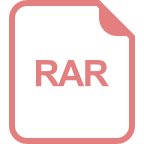
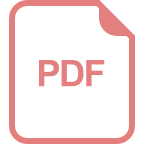
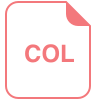
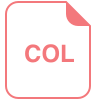
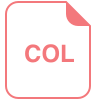
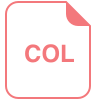
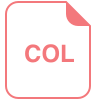









