编写C语言代码实现上述功能
时间: 2024-02-12 07:08:08 浏览: 26
好的,以下是一个简单的C语言代码实现上述功能的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/wait.h>
#define MAX_CMD_LEN 1024
#define MAX_HISTORY_LEN 100
char* history[MAX_HISTORY_LEN];
int history_len = 0;
void add_history(char* cmd) {
if (history_len == MAX_HISTORY_LEN) {
free(history[0]);
for (int i = 0; i < history_len - 1; i++) {
history[i] = history[i + 1];
}
history_len--;
}
history[history_len++] = strdup(cmd);
}
void print_history() {
for (int i = 0; i < history_len; i++) {
printf("%d\t%s\n", i + 1, history[i]);
}
}
int main(void) {
char cmd[MAX_CMD_LEN];
while (1) {
printf(">");
fflush(stdout);
// 读取用户输入
if (fgets(cmd, MAX_CMD_LEN, stdin) == NULL) {
break;
}
// 去除末尾的换行符
cmd[strlen(cmd) - 1] = '\0';
// 执行命令
if (strcmp(cmd, "exit") == 0) {
break;
} else if (strcmp(cmd, "history") == 0) {
print_history();
} else {
// 创建子进程执行命令
pid_t pid = fork();
if (pid == 0) {
// 子进程
char* args[MAX_CMD_LEN];
int argc = 0;
// 解析命令行参数
char* p = strtok(cmd, " ");
while (p != NULL) {
args[argc++] = p;
p = strtok(NULL, " ");
}
args[argc] = NULL;
// 检查是否存在重定向符号
char* infile = NULL;
char* outfile = NULL;
for (int i = 0; i < argc; i++) {
if (strcmp(args[i], "<") == 0) {
if (i < argc - 1) {
infile = args[i + 1];
args[i] = NULL;
} else {
fprintf(stderr, "syntax error near '<'\n");
exit(1);
}
} else if (strcmp(args[i], ">") == 0) {
if (i < argc - 1) {
outfile = args[i + 1];
args[i] = NULL;
} else {
fprintf(stderr, "syntax error near '>'\n");
exit(1);
}
}
}
// 重定向标准输入和标准输出
if (infile != NULL) {
freopen(infile, "r", stdin);
}
if (outfile != NULL) {
freopen(outfile, "w", stdout);
}
// 执行命令
execvp(args[0], args);
// 执行失败
fprintf(stderr, "command not found: %s\n", args[0]);
exit(1);
} else if (pid > 0) {
// 父进程
add_history(cmd);
// 等待子进程结束
waitpid(pid, NULL, 0);
} else {
perror("fork");
}
}
}
return 0;
}
```
该程序使用一个循环来读取用户输入,然后解析用户输入并执行相应的命令。在执行命令时,程序会创建一个子进程,并使用exec函数族来执行命令。如果命令中存在重定向符号,则程序会使用dup2函数将标准输入或标准输出重定向到用户指定的文件。在执行完命令后,程序会将命令添加到历史记录中,并等待子进程结束。
相关推荐
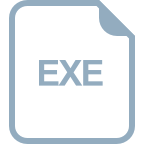
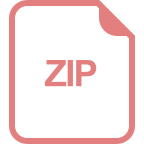
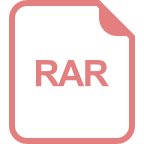














