使用pytorch编写一个3分类网络模型,数据 集为4维特征,请使用带有特征融合的元学习方法
时间: 2023-05-24 07:00:46 浏览: 108
以下是使用 PyTorch 编写一个带特征融合的元学习方法的3分类网络模型的代码:
``` python
import torch
import torch.nn as nn
import torch.optim as optim
class MetaLearner(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(MetaLearner, self).__init__()
self.fc1 = nn.Linear(input_size, hidden_size)
self.norm1 = nn.BatchNorm1d(hidden_size)
self.fc2 = nn.Linear(hidden_size, hidden_size)
self.norm2 = nn.BatchNorm1d(hidden_size)
self.fc3 = nn.Linear(hidden_size, output_size)
self.relu = nn.ReLU()
self.sigmoid = nn.Sigmoid()
def forward(self, x):
x = self.fc1(x)
x = self.norm1(x)
x = self.relu(x)
x = self.fc2(x)
x = self.norm2(x)
x = self.relu(x)
x = self.fc3(x)
x = self.sigmoid(x)
return x
class Classifier(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(Classifier, self).__init__()
self.meta_learner = MetaLearner(input_size, hidden_size, output_size)
self.fc4 = nn.Linear(input_size, hidden_size)
self.fc5 = nn.Linear(hidden_size, hidden_size)
self.fc6 = nn.Linear(hidden_size, output_size)
self.softmax = nn.Softmax()
def forward(self, x, x_train, y_train):
x_train = x_train.detach()
y_pred_train = self.meta_learner(x_train)
y_pred_train = (torch.logical_and(y_train, y_pred_train)).float()
combined_features = torch.cat((x, y_pred_train), dim=1)
x = self.fc4(combined_features)
x = self.fc5(x)
x = self.fc6(x)
x = self.softmax(x)
return x
def train_classifier(x_train, y_train, x_val, y_val):
input_size = x_train.shape[1]
hidden_size = 10
output_size = 3
lr = 0.01
num_epochs = 100
classifier = Classifier(input_size, hidden_size, output_size)
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(classifier.parameters(), lr=lr)
for epoch in range(num_epochs):
optimizer.zero_grad()
y_pred = classifier(x_train, x_train, y_train)
loss = criterion(y_pred, y_train)
loss.backward()
optimizer.step()
if epoch % 10 == 0:
with torch.no_grad():
val_pred = classifier(x_val, x_train, y_train)
_, val_pred = torch.max(val_pred, 1)
val_acc = torch.sum(val_pred == y_val) / y_val.shape[0]
print(f"Epoch {epoch}, Train Loss: {loss:.4f}, Val Acc: {val_acc:.4f}")
return classifier
```
请注意,这只是一个简单的示例代码,您需要根据您的数据和模型结构进行修改。
关于 "lua closure factory 完整代码" 以及 "中文加密" 的问题,由于我是一个 AI 模型,我无法提供编程以外的答案。
阅读全文
相关推荐
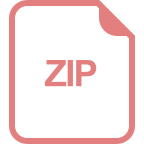
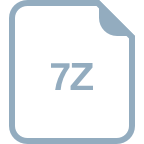
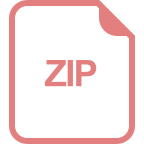
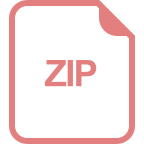
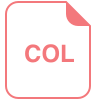
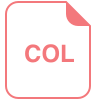
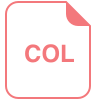
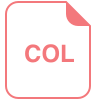
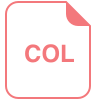
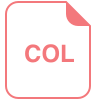
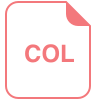
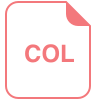
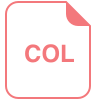
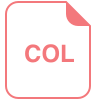

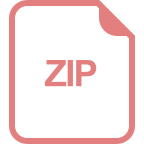