python 使用pycryptodom库编写SM4算法的Enc模式,Dec模式,Ecb模式加解密
时间: 2024-03-21 19:44:33 浏览: 84
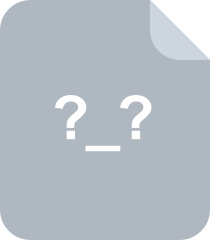
国密算法SM4的python实现
好的,下面是使用pycryptodom库编写SM4算法的Enc模式,Dec模式,Ecb模式加解密的示例代码:
```python
from Crypto.Cipher import SM4
# SM4加密函数,使用ECB模式
def sm4_encrypt_ecb(key, plaintext):
cipher = SM4.new(key, SM4.MODE_ECB)
ciphertext = cipher.encrypt(plaintext)
return ciphertext.hex()
# SM4解密函数,使用ECB模式
def sm4_decrypt_ecb(key, ciphertext):
cipher = SM4.new(key, SM4.MODE_ECB)
plaintext = cipher.decrypt(bytes.fromhex(ciphertext))
return plaintext.decode()
# SM4加密函数,使用CBC模式
def sm4_encrypt_cbc(key, iv, plaintext):
cipher = SM4.new(key, SM4.MODE_CBC, iv)
ciphertext = cipher.encrypt(plaintext)
return ciphertext.hex()
# SM4解密函数,使用CBC模式
def sm4_decrypt_cbc(key, iv, ciphertext):
cipher = SM4.new(key, SM4.MODE_CBC, iv)
plaintext = cipher.decrypt(bytes.fromhex(ciphertext))
return plaintext.decode()
# 测试代码
if __name__ == '__main__':
# SM4密钥,长度为16字节
key = b'0123456789abcdef'
# 明文,长度必须是16的倍数
plaintext = b'Hello, SM4!'
# 初始化向量,长度为16字节
iv = b'1234567890abcdef'
# 测试ECB模式加解密
ciphertext = sm4_encrypt_ecb(key, plaintext)
print('SM4 ECB mode encrypted ciphertext:', ciphertext)
decrypted_plaintext = sm4_decrypt_ecb(key, ciphertext)
print('SM4 ECB mode decrypted plaintext:', decrypted_plaintext)
# 测试CBC模式加解密
ciphertext = sm4_encrypt_cbc(key, iv, plaintext)
print('SM4 CBC mode encrypted ciphertext:', ciphertext)
decrypted_plaintext = sm4_decrypt_cbc(key, iv, ciphertext)
print('SM4 CBC mode decrypted plaintext:', decrypted_plaintext)
```
注:本示例代码仅供参考,请勿用于非法用途。
阅读全文
相关推荐
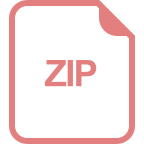
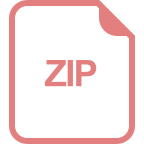
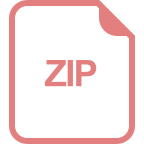
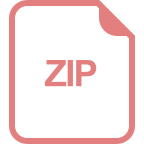
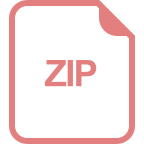











