Design and implement circle class and cylinder class. class circle must be the superclass of class cylinder. class circle cannot have any superclass except the ultimate superclass object. when instantiating the two classes , circles and cylinders can be made and stored in an array list as shown below: Arraylist<Circle> shapes=new Arraylist <Circle>(); shapes.add (new Circle());shapes.add(new Circle(5));shapes.add(new Cylinder());shapes.add(new Cylinder(10,5));for (Circle next:shapes){ System.out.println(next);} Implement both classes by including only the following members in each of the classes:instance variables,overloading consructors,getters,setters,overriding toString,overriding equals
时间: 2024-03-18 10:38:39 浏览: 83
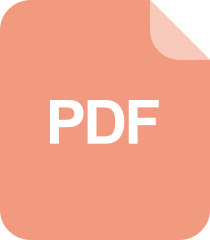
类point、类circl、类cylinder的程序设计[归纳].pdf
Sure, I can help you with that. Here is the implementation of the Circle and Cylinder classes:
```java
public class Circle {
private double radius;
public Circle() {
this.radius = 0.0;
}
public Circle(double radius) {
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
@Override
public String toString() {
return "Circle with radius " + radius;
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Circle)) {
return false;
}
Circle other = (Circle) obj;
return Double.compare(radius, other.radius) == 0;
}
}
public class Cylinder extends Circle {
private double height;
public Cylinder() {
super();
this.height = 0.0;
}
public Cylinder(double radius, double height) {
super(radius);
this.height = height;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
@Override
public String toString() {
return "Cylinder with radius " + getRadius() + " and height " + height;
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Cylinder)) {
return false;
}
Cylinder other = (Cylinder) obj;
return super.equals(other) && Double.compare(height, other.height) == 0;
}
}
```
In this implementation, the Circle class has only one instance variable - `radius`, and the Cylinder class has an additional instance variable - `height`. Both classes have two constructors each - one with no arguments and one with `radius` argument for Circle and `radius` and `height` arguments for Cylinder. Both classes have getter and setter methods for their instance variables. The `toString()` method is overridden to return a string representation of the object, and the `equals()` method is overridden to compare two objects based on their instance variables.
You can use these classes as shown in the code snippet you provided, to create circles and cylinders and store them in an ArrayList of Circles.
阅读全文
相关推荐
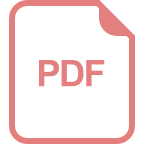
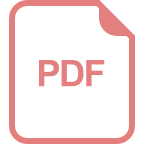

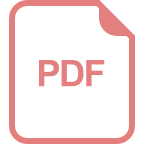
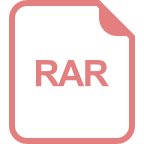
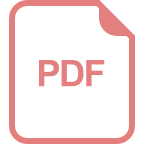
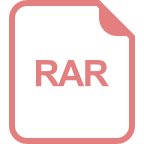
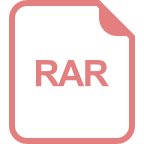
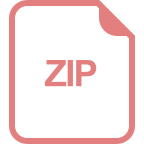
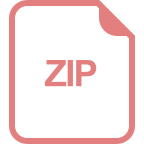
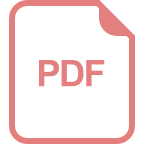
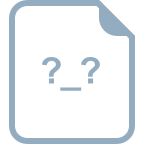
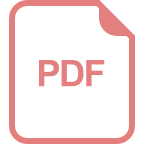
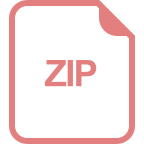
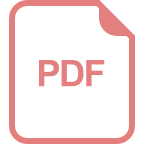
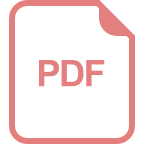