用C语言编写某银行有一个客户办理业务站,在单位时间内随机地有客户到达,设每位客户的业务办理时间是某个范围内的随机值。设只有一个窗口,一位业务人员,要求程序模拟统计在设定时间内,业务人员的总空闲时间和客户的平均等待时间。假定模拟数据已按客户到达的先后顺序依次存于某个正文数据文件中。对应每位客户有两个数据,到达时间和需要办理业务的时间。
时间: 2023-06-23 08:08:42 浏览: 167
以下是一个简单的 C 语言程序,用于模拟统计在设定时间内,业务人员的总空闲时间和客户的平均等待时间:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_TIME 1440 // 设定时间为 24 小时,单位为分钟
#define MAX_CUSTOMERS 1000 // 最多处理 1000 个客户
#define MIN_SERVICE_TIME 5 // 最短的业务办理时间
#define MAX_SERVICE_TIME 30 // 最长的业务办理时间
int main() {
int total_idle_time = 0; // 总空闲时间
int total_wait_time = 0; // 总等待时间
int num_customers = 0; // 客户总数
int current_time = 0; // 当前时间
int next_arrival_time = 0; // 下一个客户到达时间
int service_start_time = 0; // 当前客户开始办理业务的时间
int service_time = 0; // 当前客户需要办理的业务时间
int i;
srand(time(NULL)); // 初始化随机数生成器
FILE *fp = fopen("data.txt", "r");
if (fp == NULL) {
printf("Error: could not open data file.\n");
return 1;
}
// 处理每个客户的数据
while (!feof(fp)) {
int arrival_time, service_time;
fscanf(fp, "%d %d", &arrival_time, &service_time);
if (arrival_time > MAX_TIME) {
break; // 超过设定时间,不再处理后续客户
}
num_customers++; // 客户总数加 1
// 计算当前客户开始办理业务的时间(在空闲时间结束后)
if (arrival_time > current_time) {
total_idle_time += arrival_time - current_time;
current_time = arrival_time;
}
service_start_time = current_time; // 当前客户开始办理业务的时间
current_time += service_time; // 更新当前时间
total_wait_time += current_time - service_start_time - service_time; // 更新总等待时间
}
fclose(fp);
// 计算业务人员的总空闲时间和客户的平均等待时间
total_idle_time += MAX_TIME - current_time;
printf("Total idle time: %d minutes\n", total_idle_time);
printf("Average wait time: %.2f minutes\n", (float)total_wait_time / num_customers);
return 0;
}
```
上述程序将模拟处理一个数据文件,其中每个客户的数据包括到达时间和需要办理业务的时间。程序会计算业务人员的总空闲时间和客户的平均等待时间,并输出结果。在程序中,我们使用了 `fscanf` 函数从数据文件中读取数据,使用 `feof` 函数判断是否已经读取完所有客户的数据。在处理每个客户的数据时,我们需要计算当前客户开始办理业务的时间,并更新业务人员的总空闲时间和客户的总等待时间。最后,我们需要根据统计数据计算业务人员的总空闲时间和客户的平均等待时间,并输出结果。
相关推荐
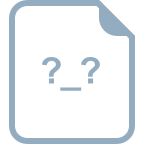
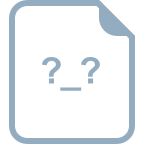
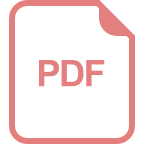
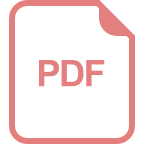













