使用java编写一个方法,参数是文件名,实现读取txt文件,使用逗号,分号,空格,回车将文件内容分割成一个一个的词组,找出所有重复的词组
时间: 2024-05-15 21:16:31 浏览: 155
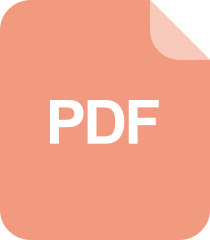
JAVA实现读取txt文件内容的方法

以下是一个可能的实现:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class DuplicateWordsFinder {
public static Set<String> findDuplicateWords(String fileName) throws IOException {
Set<String> allWords = new HashSet<>();
Set<String> duplicateWords = new HashSet<>();
BufferedReader reader = new BufferedReader(new FileReader(fileName));
String line;
while ((line = reader.readLine()) != null) {
// 使用正则表达式将每行内容分割成词组
String[] wordsInLine = line.split("[,;\\s]+");
for (String word : wordsInLine) {
if (!word.isEmpty()) { // 忽略空字符串
if (allWords.contains(word)) {
duplicateWords.add(word);
} else {
allWords.add(word);
}
}
}
}
reader.close();
return duplicateWords;
}
public static void main(String[] args) {
try {
Set<String> duplicateWords = findDuplicateWords("example.txt");
if (duplicateWords.isEmpty()) {
System.out.println("No duplicate words found.");
} else {
System.out.print("Duplicate words found: ");
for (String word : duplicateWords) {
System.out.print(word + " ");
}
System.out.println();
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
}
```
这个方法的思路是:
1. 用一个 `HashSet` 来存储所有出现过的词组。
2. 遍历文件的每一行,使用正则表达式将每行内容分割成词组。
3. 对于每个词组,如果它已经在 `allWords` 中出现过了,就把它加到 `duplicateWords` 中;否则,把它加到 `allWords` 中。
4. 返回 `duplicateWords`。
`main` 方法调用了 `findDuplicateWords` 方法,打印出找到的重复词组。注意,如果文件不存在或无法读取,方法会抛出 `IOException`,需要在调用时进行处理。
这个方法可能存在一些限制和缺陷,比如:
- 词组中可能包含标点符号或其他特殊字符,这会影响词组的判断。
- 词组中可能包含大小写不同的版本,这会导致重复词组未被发现。
- 如果文件很大,一次性读取可能会导致内存不足。
- 如果文件编码方式不是 UTF-8,可能会出现乱码。
阅读全文
相关推荐
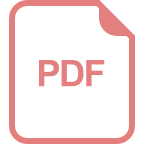
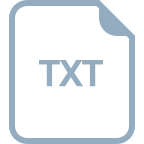
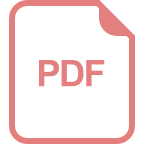
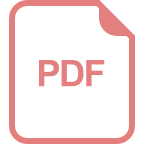
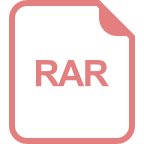
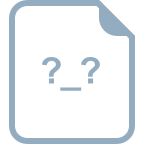
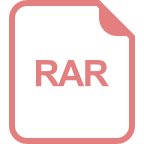
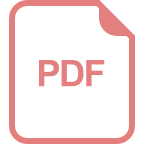
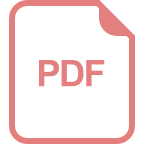
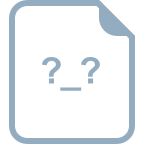
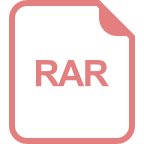