#include <iostream.h> class Matrix { public: Matrix(int r, int c) {} row = r; col = c; elem = new double[row * col]; double& operator( )(int x, int y) { return elem[x * col + y]; } ~Matrix() { delete[] elem; } private: double* elem; int row, col; }; void main() { Matrix m(5, 8); for (int i = 0; i < 5; i++) { m(i, 2) = i + 15; cout << m(i, 2) << ","; } cout << endl; }
时间: 2024-01-22 22:03:58 浏览: 64
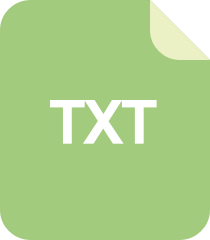
#include"iostream.h"

这段代码有一些语法错误:
1. `<iostream.h>` 库已经过时,正确的头文件应该是 `<iostream>`。
2. `Matrix` 类的构造函数定义不正确,应该将花括号移到 `row` 和 `col` 的赋值语句之前。
3. `Matrix` 类的 `operator()` 函数定义不正确,应该将大括号移到函数头之后。
以下是修改后的代码:
```c++
#include <iostream>
using namespace std;
class Matrix {
public:
Matrix(int r, int c) {
row = r;
col = c;
elem = new double[row * col];
}
double& operator()(int x, int y) {
return elem[x * col + y];
}
~Matrix() {
delete[] elem;
}
private:
double* elem;
int row, col;
};
int main() {
Matrix m(5, 8);
for (int i = 0; i < 5; i++) {
m(i, 2) = i + 15;
cout << m(i, 2) << ",";
}
cout << endl;
return 0;
}
```
修改后的代码可以正确地创建一个 $5 \times 8$ 的矩阵对象 `m`,并对其中的元素进行读写操作。运行结果如下:
```
15,16,17,18,19,
```
阅读全文
相关推荐
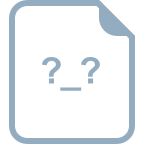


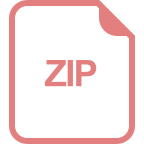
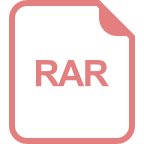











