编写程序实现购物车商品自定义单价,并计算总价。
时间: 2024-03-02 11:51:49 浏览: 26
以下是一个简单的示例代码,可以让用户自定义商品的单价并计算购物车的总价。代码使用了HTML、CSS和JavaScript,使用了表单元素和事件处理等基础知识。
```html
<!DOCTYPE html>
<html>
<head>
<title>购物车示例</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
border: 1px solid black;
padding: 8px;
text-align: center;
}
th {
background-color: #ddd;
font-weight: bold;
}
.totalPrice {
font-weight: bold;
margin-top: 10px;
}
</style>
<script>
function calculateTotal() {
// 获取表格中所有单价输入框和数量输入框
var priceInputs = document.querySelectorAll(".priceInput");
var quantityInputs = document.querySelectorAll(".quantityInput");
var total = 0;
for (var i = 0; i < priceInputs.length; i++) {
var price = parseFloat(priceInputs[i].value);
var quantity = parseInt(quantityInputs[i].value);
if (!isNaN(price) && !isNaN(quantity)) {
total += price * quantity;
}
}
// 将总价显示在页面上
document.getElementById("totalPrice").innerHTML = "总价:" + total.toFixed(2);
}
</script>
</head>
<body>
<h1>购物车</h1>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>单价</th>
<th>数量</th>
</tr>
</thead>
<tbody>
<tr>
<td>商品1</td>
<td><input type="number" class="priceInput" value="10"></td>
<td><input type="number" class="quantityInput" value="1"></td>
</tr>
<tr>
<td>商品2</td>
<td><input type="number" class="priceInput" value="15"></td>
<td><input type="number" class="quantityInput" value="1"></td>
</tr>
<tr>
<td>商品3</td>
<td><input type="number" class="priceInput" value="20"></td>
<td><input type="number" class="quantityInput" value="1"></td>
</tr>
</tbody>
</table>
<button onclick="calculateTotal()">计算总价</button>
<p id="totalPrice" class="totalPrice"></p>
</body>
</html>
```
在代码中,我们先定义了一个表格,其中每个商品的单价和数量都是通过输入框进行输入的。我们给单价输入框和数量输入框都加上了相应的类名,以便在JavaScript代码中获取它们的值。
在JavaScript代码中,我们定义了一个calculateTotal函数,用于计算购物车的总价。函数首先获取表格中所有单价输入框和数量输入框,然后遍历它们计算出总价,并将总价显示在页面上。
在HTML代码中,我们添加了一个计算总价的按钮和一个用于显示总价的p元素。点击按钮时会调用calculateTotal函数计算总价,并将总价显示在页面上。
相关推荐
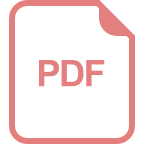














